springboot自定义jackson
时间: 2023-08-29 19:13:30 浏览: 111
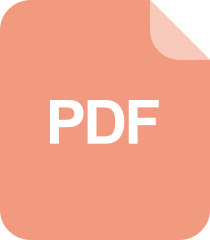
SpringBoot使用自定义json解析器的使用方法
在Spring Boot中,可以通过自定义Jackson来实现自定义序列化和反序列化的需求。以下是一个简单的示例,演示了如何自定义Jackson。
首先,我们需要创建一个Jackson的ObjectMapper Bean,并指定我们自定义的序列化器和反序列化器。示例中,我们自定义了一个格式化日期的序列化器和反序列化器。
```java
@Configuration
public class JacksonConfig {
@Bean
public ObjectMapper objectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
SimpleModule module = new SimpleModule();
module.addSerializer(Date.class, new CustomDateSerializer());
module.addDeserializer(Date.class, new CustomDateDeserializer());
objectMapper.registerModule(module);
return objectMapper;
}
}
```
然后,我们需要实现自定义的序列化器和反序列化器。示例中,我们自定义了一个格式化日期的序列化器和反序列化器。
```java
public class CustomDateSerializer extends JsonSerializer<Date> {
private static final SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
@Override
public void serialize(Date value, JsonGenerator gen, SerializerProvider serializers) throws IOException {
gen.writeString(dateFormat.format(value));
}
}
public class CustomDateDeserializer extends JsonDeserializer<Date> {
private static final SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
@Override
public Date deserialize(JsonParser p, DeserializationContext ctxt) throws IOException {
String dateValue = p.getText();
try {
return dateFormat.parse(dateValue);
} catch (ParseException e) {
throw new RuntimeException(e);
}
}
}
```
现在,我们已经完成了自定义Jackson的配置。在使用时,我们可以直接使用@Autowired注解注入ObjectMapper Bean,或者使用@JsonComponent注解来标识我们的自定义序列化器和反序列化器。
```java
@RestController
public class UserController {
@Autowired
private ObjectMapper objectMapper;
@PostMapping("/user")
public User addUser(@RequestBody User user) throws JsonProcessingException {
String json = objectMapper.writeValueAsString(user);
System.out.println(json);
return user;
}
}
@JsonComponent
public class DateJsonComponent {
public static class Serializer extends JsonSerializer<Date> {
private static final SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
@Override
public void serialize(Date value, JsonGenerator gen, SerializerProvider serializers) throws IOException {
gen.writeString(dateFormat.format(value));
}
}
public static class Deserializer extends JsonDeserializer<Date> {
private static final SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
@Override
public Date deserialize(JsonParser p, DeserializationContext ctxt) throws IOException {
String dateValue = p.getText();
try {
return dateFormat.parse(dateValue);
} catch (ParseException e) {
throw new RuntimeException(e);
}
}
}
}
```
以上就是自定义Jackson的简单示例。通过自定义Jackson,我们可以轻松实现自定义的序列化和反序列化需求。
阅读全文
相关推荐
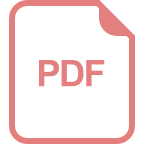
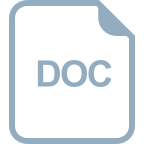
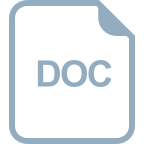

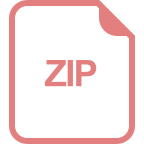
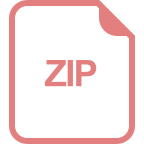
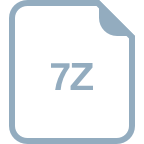
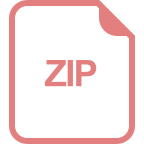
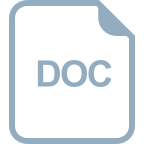



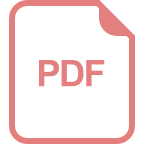
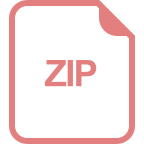
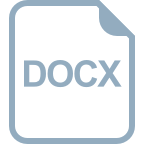
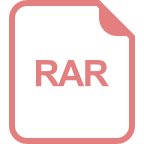
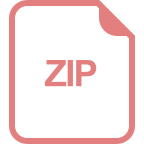
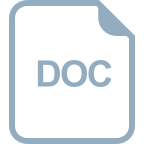