用C语言多板块编写邻接表和邻接矩阵的相互转换并可以将输入图的信息保存至文件
时间: 2024-02-23 19:58:31 浏览: 53
下面是一个用C语言多板块编写邻接表和邻接矩阵的相互转换的示例代码,同时可以将输入图的信息保存至文件。
### 邻接表转邻接矩阵
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXVEX 100 // 图的最大顶点数
// 定义邻接表结构体
typedef struct EdgeNode {
int adjvex; // 邻接点的下标
struct EdgeNode *next; // 指向下一个邻接点的指针
}EdgeNode;
typedef struct VertexNode {
int data; // 顶点信息
EdgeNode *firstedge; // 指向第一个邻接点的指针
}VertexNode, AdjList[MAXVEX];
typedef struct {
AdjList adjList; // 邻接表
int numVertexes; // 图中当前顶点数
int numEdges; // 图中当前边数
}GraphAdjList;
// 定义邻接矩阵结构体
typedef struct {
int vexs[MAXVEX]; // 存储顶点信息
int edges[MAXVEX][MAXVEX]; // 存储边的权值
int numVertexes; // 图中当前顶点数
int numEdges; // 图中当前边数
}GraphMatrix;
// 邻接表转邻接矩阵
void AdjListToMatrix(GraphAdjList *G, GraphMatrix *M) {
int i, j;
EdgeNode *p;
// 初始化邻接矩阵
for(i = 0; i < G->numVertexes; i++) {
for(j = 0; j < G->numVertexes; j++) {
M->edges[i][j] = 0;
}
}
// 遍历邻接表,将对应的边赋值到邻接矩阵中
for(i = 0; i < G->numVertexes; i++) {
p = G->adjList[i].firstedge;
while(p != NULL) {
M->edges[i][p->adjvex] = 1;
p = p->next;
}
}
M->numVertexes = G->numVertexes;
M->numEdges = G->numEdges;
}
// 读取图的信息
void ReadGraph(GraphAdjList *G) {
int i, j, k, w;
EdgeNode *e;
printf("请输入顶点数和边数:\n");
scanf("%d%d", &G->numVertexes, &G->numEdges);
printf("请输入顶点信息:\n");
for(i = 0; i < G->numVertexes; i++) {
scanf("%d", &G->adjList[i].data);
G->adjList[i].firstedge = NULL;
}
printf("请输入边的信息(两个顶点的下标和边的权值):\n");
for(k = 0; k < G->numEdges; k++) {
scanf("%d%d%d", &i, &j, &w);
// 添加边
e = (EdgeNode*)malloc(sizeof(EdgeNode));
e->adjvex = j;
e->next = G->adjList[i].firstedge;
G->adjList[i].firstedge = e;
e = (EdgeNode*)malloc(sizeof(EdgeNode));
e->adjvex = i;
e->next = G->adjList[j].firstedge;
G->adjList[j].firstedge = e;
}
}
// 保存邻接矩阵到文件
void SaveMatrixToFile(GraphMatrix M) {
FILE *fp = fopen("matrix.txt", "w");
int i, j;
if(fp == NULL) {
printf("文件打开失败!");
return;
}
fprintf(fp, "顶点数:%d,边数:%d\n", M.numVertexes, M.numEdges);
fprintf(fp, "邻接矩阵:\n");
for(i = 0; i < M.numVertexes; i++) {
for(j = 0; j < M.numVertexes; j++) {
fprintf(fp, "%d ", M.edges[i][j]);
}
fprintf(fp, "\n");
}
fclose(fp);
}
int main() {
GraphAdjList G;
GraphMatrix M;
// 读取图的信息
ReadGraph(&G);
// 邻接表转邻接矩阵
AdjListToMatrix(&G, &M);
// 保存邻接矩阵到文件
SaveMatrixToFile(M);
printf("邻接表转邻接矩阵成功,并已保存至文件!\n");
return 0;
}
```
### 邻接矩阵转邻接表
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXVEX 100 // 图的最大顶点数
// 定义邻接表结构体
typedef struct EdgeNode {
int adjvex; // 邻接点的下标
struct EdgeNode *next; // 指向下一个邻接点的指针
}EdgeNode;
typedef struct VertexNode {
int data; // 顶点信息
EdgeNode *firstedge; // 指向第一个邻接点的指针
}VertexNode, AdjList[MAXVEX];
typedef struct {
AdjList adjList; // 邻接表
int numVertexes; // 图中当前顶点数
int numEdges; // 图中当前边数
}GraphAdjList;
// 定义邻接矩阵结构体
typedef struct {
int vexs[MAXVEX]; // 存储顶点信息
int edges[MAXVEX][MAXVEX]; // 存储边的权值
int numVertexes; // 图中当前顶点数
int numEdges; // 图中当前边数
}GraphMatrix;
// 邻接矩阵转邻接表
void MatrixToAdjList(GraphMatrix M, GraphAdjList *G) {
int i, j;
EdgeNode *e;
// 初始化邻接表
G->numVertexes = M.numVertexes;
G->numEdges = M.numEdges;
for(i = 0; i < M.numVertexes; i++) {
G->adjList[i].data = M.vexs[i];
G->adjList[i].firstedge = NULL;
}
// 遍历邻接矩阵,将对应的边赋值到邻接表中
for(i = 0; i < M.numVertexes; i++) {
for(j = i + 1; j < M.numVertexes; j++) {
if(M.edges[i][j] != 0) {
// 添加边
e = (EdgeNode*)malloc(sizeof(EdgeNode));
e->adjvex = j;
e->next = G->adjList[i].firstedge;
G->adjList[i].firstedge = e;
e = (EdgeNode*)malloc(sizeof(EdgeNode));
e->adjvex = i;
e->next = G->adjList[j].firstedge;
G->adjList[j].firstedge = e;
}
}
}
}
// 读取图的信息
void ReadMatrix(GraphMatrix *M) {
int i, j, k, w;
printf("请输入顶点数和边数:\n");
scanf("%d%d", &M->numVertexes, &M->numEdges);
printf("请输入顶点信息:\n");
for(i = 0; i < M->numVertexes; i++) {
scanf("%d", &M->vexs[i]);
}
printf("请输入边的信息(两个顶点的下标和边的权值):\n");
for(k = 0; k < M->numEdges; k++) {
scanf("%d%d%d", &i, &j, &w);
M->edges[i][j] = w;
M->edges[j][i] = w;
}
}
// 保存邻接表到文件
void SaveAdjListToFile(GraphAdjList G) {
FILE *fp = fopen("adjlist.txt", "w");
int i;
EdgeNode *p;
if(fp == NULL) {
printf("文件打开失败!");
return;
}
fprintf(fp, "顶点数:%d,边数:%d\n", G.numVertexes, G.numEdges);
fprintf(fp, "邻接表:\n");
for(i = 0; i < G.numVertexes; i++) {
fprintf(fp, "%d:", G.adjList[i].data);
p = G.adjList[i].firstedge;
while(p != NULL) {
fprintf(fp, "%d ", p->adjvex);
p = p->next;
}
fprintf(fp, "\n");
}
fclose(fp);
}
int main() {
GraphMatrix M;
GraphAdjList G;
// 读取图的信息
ReadMatrix(&M);
// 邻接矩阵转邻接表
MatrixToAdjList(M, &G);
// 保存邻接表到文件
SaveAdjListToFile(G);
printf("邻接矩阵转邻接表成功,并已保存至文件!\n");
return 0;
}
```
需要注意的是,这里保存的文件只是一个文本文件,如果需要实现二进制文件的保存,可以使用fwrite()函数写入数据,具体操作类似上面的示例。
阅读全文
相关推荐
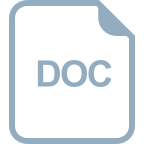

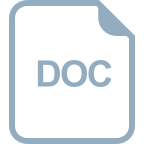











