用Python编写描述 输入一个中文句子,以回车结束。统计并输出句子中字符个数及中文词数,要求中文分司采用jeba库中的Icut()函数进输入格式 输入一个中文句子,以回车结束 输出格式 字符个数及中文词数
时间: 2024-02-24 17:57:21 浏览: 250
好的,以下是代码实现:
```python
import jieba
# 输入句子
sentence = input("请输入一个中文句子,以回车结束:")
# 统计字符个数
char_num = len(sentence)
# 分词并统计中文词数
word_list = jieba.cut(sentence)
cn_word_num = 0
for word in word_list:
if len(word) > 1:
cn_word_num += 1
# 输出结果
print("字符个数为:", char_num)
print("中文词数为:", cn_word_num)
```
注意,需要提前安装 jieba 库,可以使用以下命令进行安装:
```
pip install jieba
```
另外,为了准确统计中文词数,上述代码中对分词结果进行了一些处理,具体实现可以参考 jieba 库的文档。
相关问题
Python,编写程序,用户输入一个以回车结束的字符串,统计字符串里英文字母、数字字符和其他字符的个数(回车符代表结束,不计入统计)
Python中,你可以通过内置函数`str.isalnum()`和`str.isdigit()`来分别检查每个字符是否是字母或数字,然后使用循环遍历用户的输入,对每一类字符进行计数。下面是一个简单的示例:
```python
def count_characters(user_input):
# 初始化计数器
letter_count = 0
digit_count = 0
other_count = 0
for char in user_input:
if char.isalpha(): # 检查是否为字母
letter_count += 1
elif char.isdigit(): # 检查是否为数字
digit_count += 1
else: # 其他字符(非字母和数字)
other_count += 1
return letter_count, digit_count, other_count
# 获取用户输入
user_string = input("请输入一个以回车结束的字符串: ")
# 调用函数并打印结果
letter, digit, other = count_characters(user_string)
print(f"英文字母数量: {letter}")
print(f"数字字符数量: {digit}")
print(f"其他字符数量: {other}")
编写程序,统计并输出某给定字符在给定字符串中出现的次数。要求使用字典实现。 输入 第一行给出一个以回车结束的字符串(少于80个字符);第二行输入一个字符。 输出 在一行中输出给定字符在给定字符串中出现的次数。
以下是 Python 代码实现:
```python
s = input() # 输入字符串
c = input() # 输入字符
d = {} # 定义空字典
for i in s:
if i in d:
d[i] += 1 # 字符已存在,计数器加1
else:
d[i] = 1 # 字符不存在,计数器设为1
if c in d:
print(d[c]) # 输出给定字符出现的次数
else:
print(0) # 给定字符不存在,输出0
```
输入示例:
```
hello world
l
```
输出示例:
```
3
```
阅读全文
相关推荐
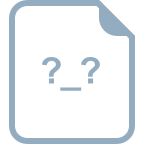













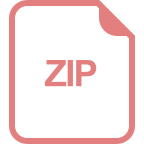