用java申明一个银行账户类,成员变量包括账号、储户姓名、开户时间、身份证号码、存款余额等账户信息,成员方法包括开户、存款、取款、查询(余额,明细)、销户等操作。
时间: 2023-07-04 08:05:42 浏览: 189
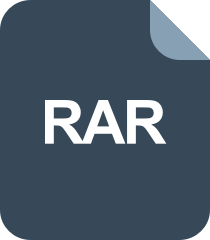
JAVA实现银行账户类

以下是一个Java代码示例:
```java
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BankAccount {
private String accountNumber; // 账号
private String accountHolderName; // 储户姓名
private Date openDate; // 开户时间
private String identityCardNumber; // 身份证号码
private double balance; // 存款余额
private List<Transaction> transactions; // 交易明细列表
public BankAccount(String accountNumber, String accountHolderName, String identityCardNumber) {
this.accountNumber = accountNumber;
this.accountHolderName = accountHolderName;
this.identityCardNumber = identityCardNumber;
this.openDate = new Date(); // 开户时间为当前时间
this.balance = 0;
this.transactions = new ArrayList<>();
}
// 存款
public void deposit(double amount) {
balance += amount;
transactions.add(new Transaction("Deposit", amount));
}
// 取款
public void withdraw(double amount) throws InsufficientFundsException {
if (amount <= balance) {
balance -= amount;
transactions.add(new Transaction("Withdrawal", -amount));
} else {
throw new InsufficientFundsException();
}
}
// 查询余额
public double getBalance() {
return balance;
}
// 查询交易明细
public List<Transaction> getTransactions() {
return transactions;
}
// 销户
public void closeAccount() {
balance = 0;
transactions.clear();
}
// 开户
public static BankAccount openAccount(String accountNumber, String accountHolderName, String identityCardNumber) {
return new BankAccount(accountNumber, accountHolderName, identityCardNumber);
}
// 交易明细类
private static class Transaction {
private String description; // 描述(存款、取款)
private double amount; // 交易金额
private Date date; // 交易时间
public Transaction(String description, double amount) {
this.description = description;
this.amount = amount;
this.date = new Date(); // 交易时间为当前时间
}
public String getDescription() {
return description;
}
public double getAmount() {
return amount;
}
public Date getDate() {
return date;
}
}
// 资金不足异常类
private static class InsufficientFundsException extends Exception {
public InsufficientFundsException() {
super("Insufficient Funds");
}
}
}
```
使用示例:
```java
// 开户
BankAccount account = BankAccount.openAccount("1234567890", "张三", "123456789012345678");
// 存款
account.deposit(1000);
// 取款
try {
account.withdraw(500);
} catch (InsufficientFundsException e) {
System.out.println(e.getMessage());
}
// 查询余额
System.out.println("账户余额:" + account.getBalance());
// 查询交易明细
List<BankAccount.Transaction> transactions = account.getTransactions();
for (BankAccount.Transaction transaction : transactions) {
System.out.println(transaction.getDescription() + " " + transaction.getAmount() + " " + transaction.getDate());
}
// 销户
account.closeAccount();
```
阅读全文
相关推荐
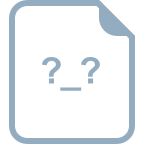













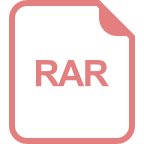