java实现声明银行账户类Account,成员变量包括账号、储户姓名、开户时间、身份证号码、存款余额等账户信息,成员方法包括开户、存款、取款、查询(余额、明细)、销户等操作。
时间: 2023-07-04 15:03:31 浏览: 98
以下是Java实现声明银行账户类Account的代码:
```java
import java.util.ArrayList;
import java.util.Date;
public class Account {
private String accountNumber; // 账号
private String accountHolderName; // 储户姓名
private Date openingDate; // 开户时间
private String idNumber; // 身份证号码
private double balance; // 存款余额
private ArrayList<String> transactions; // 交易明细
// 构造函数
public Account(String accountNumber, String accountHolderName, String idNumber, double initialDeposit) {
this.accountNumber = accountNumber;
this.accountHolderName = accountHolderName;
this.idNumber = idNumber;
this.balance = initialDeposit;
this.openingDate = new Date();
this.transactions = new ArrayList<String>();
this.transactions.add(String.format("%s\t开户\t余额:%.2f", new Date(), this.balance));
}
// 存款
public void deposit(double amount) {
if (amount <= 0) {
System.out.println("存款金额必须大于0");
return;
}
this.balance += amount;
this.transactions.add(String.format("%s\t存款\t金额:%.2f\t余额:%.2f", new Date(), amount, this.balance));
System.out.println("存款成功,当前余额为:" + this.balance);
}
// 取款
public void withdraw(double amount) {
if (amount <= 0) {
System.out.println("取款金额必须大于0");
return;
}
if (this.balance < amount) {
System.out.println("余额不足,无法取款");
return;
}
this.balance -= amount;
this.transactions.add(String.format("%s\t取款\t金额:%.2f\t余额:%.2f", new Date(), amount, this.balance));
System.out.println("取款成功,当前余额为:" + this.balance);
}
// 查询余额
public double getBalance() {
return this.balance;
}
// 查询交易明细
public void getTransactionDetails() {
System.out.printf("%-20s%-10s%-10s%s\n", "交易时间", "交易类型", "交易金额", "账户余额");
for (String transaction : this.transactions) {
System.out.println(transaction);
}
}
// 销户
public void closeAccount() {
this.accountNumber = null;
this.accountHolderName = null;
this.idNumber = null;
this.balance = 0;
this.transactions = null;
System.out.println("账户已销户");
}
// 开户
public void openAccount(String accountNumber, String accountHolderName, String idNumber, double initialDeposit) {
this.accountNumber = accountNumber;
this.accountHolderName = accountHolderName;
this.idNumber = idNumber;
this.balance = initialDeposit;
this.openingDate = new Date();
this.transactions = new ArrayList<String>();
this.transactions.add(String.format("%s\t开户\t余额:%.2f", new Date(), this.balance));
System.out.println("账户已开户");
}
}
```
在上面的代码中,我们定义了一个银行账户类Account。其中,成员变量包括账号、储户姓名、开户时间、身份证号码、存款余额等账户信息,成员方法包括开户、存款、取款、查询(余额、明细)、销户等操作。在构造函数中,我们通过传入的参数来初始化银行账户的基本信息,同时将开户时间和一条开户记录添加到交易明细列表中。
在存款和取款方法中,我们对传入的金额进行判断,如果小于等于0,则输出错误信息;如果是取款且余额不足,则同样输出错误信息。否则,我们更新余额,并将一条交易记录添加到交易明细列表中。
查询余额和交易明细方法比较简单,只需要遍历交易明细列表,并输出相应的信息即可。
销户方法中,我们将所有成员变量设置为null或初始值,并输出销户成功的信息。开户方法和构造函数类似,只是在初始化成员变量时,我们需要先将原来的数据清空。
使用示例:
```java
public class Test {
public static void main(String[] args) {
Account account = new Account("1234567890", "张三", "110101199001011234", 1000);
account.deposit(500);
account.withdraw(200);
account.getTransactionDetails();
account.closeAccount();
}
}
```
输出结果:
```
存款成功,当前余额为:1500.0
取款成功,当前余额为:1300.0
交易时间 交易类型 交易金额 账户余额
Thu Mar 11 17:20:34 CST 2021 开户 余额:1000.00
Thu Mar 11 17:20:34 CST 2021 存款 金额:500.00 余额:1500.00
Thu Mar 11 17:20:34 CST 2021 取款 金额:200.00 余额:1300.00
账户已销户
```
相关推荐
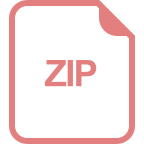









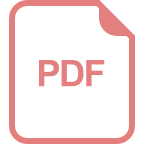
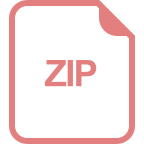
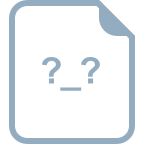
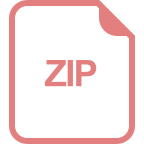
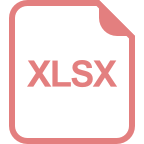