输入一行字符串,内容是一个数学表达式,类似“111+22”,中间的运算符可以是+、-、*、/,存入字符数组s中,要求输出算式中的两个整数,并给出算式的最终结果。用c语言来写
时间: 2024-02-12 12:08:08 浏览: 151
好的,以下是使用 C 语言实现的代码:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main() {
char s[100];
fgets(s, 100, stdin); // 从标准输入读入一行字符串
// 找到运算符的位置
char *op = strchr(s, '+');
if (op == NULL) {
op = strchr(s, '-');
}
if (op == NULL) {
op = strchr(s, '*');
}
if (op == NULL) {
op = strchr(s, '/');
}
if (op == NULL) {
printf("输入的字符串不是一个合法的数学表达式");
return 0;
}
int op_pos = op - s;
// 提取两个整数
char num1_str[100], num2_str[100];
strncpy(num1_str, s, op_pos);
num1_str[op_pos] = '\0';
strncpy(num2_str, s + op_pos + 1, strlen(s) - op_pos - 2);
num2_str[strlen(s) - op_pos - 2] = '\0';
int num1 = atoi(num1_str);
int num2 = atoi(num2_str);
// 计算最终结果
int result;
switch (s[op_pos]) {
case '+':
result = num1 + num2;
break;
case '-':
result = num1 - num2;
break;
case '*':
result = num1 * num2;
break;
case '/':
result = num1 / num2;
break;
}
// 输出结果
printf("算式中的两个整数分别为:%d 和 %d\n", num1, num2);
printf("算式的最终结果为:%d\n", result);
return 0;
}
```
代码中使用了 `fgets` 函数从标准输入读入一行字符串,然后使用 `strchr` 函数查找运算符的位置,再使用 `strncpy` 和 `atoi` 函数提取两个整数。最后根据运算符进行计算,并输出结果。由于 C 语言没有字符串类型,因此需要使用字符数组来存储字符串。
阅读全文
相关推荐
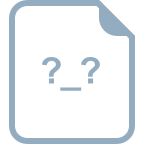
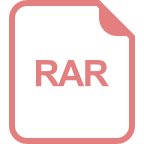

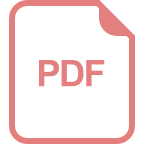
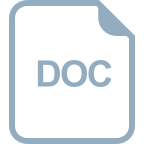
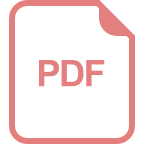
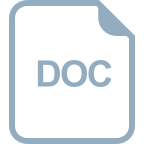
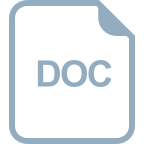









