定义数组arr,值为[‘a’, ‘b’, ‘b’, ‘c’, ‘d’, ’d’],统计重复的字符有多少个,几种方法
时间: 2023-09-27 07:05:01 浏览: 30
有以下几种方法可以统计重复的字符数量:
1. 使用Counter类
```python
from collections import Counter
arr = ['a', 'b', 'b', 'c', 'd', 'd']
counter = Counter(arr)
# 统计重复的字符数量
duplicate_count = sum([count - 1 for count in counter.values() if count > 1])
print(duplicate_count) # 3
# 统计重复的字符种类数量
duplicate_type_count = len([count for count in counter.values() if count > 1])
print(duplicate_type_count) # 2
```
2. 使用set和count方法
```python
arr = ['a', 'b', 'b', 'c', 'd', 'd']
duplicate_count = sum([arr.count(x) - 1 for x in set(arr) if arr.count(x) > 1])
print(duplicate_count) # 3
duplicate_type_count = len([x for x in set(arr) if arr.count(x) > 1])
print(duplicate_type_count) # 2
```
3. 使用for循环和字典
```python
arr = ['a', 'b', 'b', 'c', 'd', 'd']
count_dict = {}
# 统计每个字符出现的次数
for char in arr:
if char not in count_dict:
count_dict[char] = 0
count_dict[char] += 1
# 统计重复的字符数量
duplicate_count = sum([count - 1 for count in count_dict.values() if count > 1])
print(duplicate_count) # 3
# 统计重复的字符种类数量
duplicate_type_count = len([count for count in count_dict.values() if count > 1])
print(duplicate_type_count) # 2
```
相关推荐
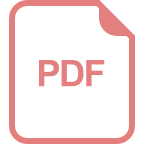
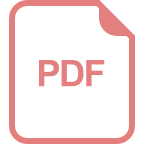
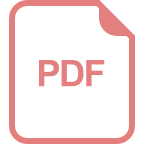
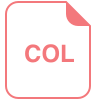
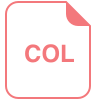
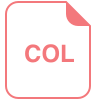
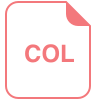
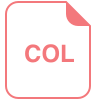









