用c语言实现统计一个给定字符串中指定的字符出现的次数 输入描述 测试输入包含若干测试用例,每个测试用例包含2行,第1行为一个长度不超过5的字符串,第2行为一个长度不超过80的字符串。注意这里的字符串包含空格,即空格也可能是要求被统计的字符之一。当读到’#'时输入结束,相应的结果不要输出。 输出描述 对每个测试用例,统计第1行中字符串的每个字符在第2行字符串中出现的次数,按如下格式输出:其中ci是第1行中第i个字符,ni是ci出现的次数。
时间: 2023-06-02 22:06:00 浏览: 94
\0’时输入结束。输出描述 对于每个测试用例,输出该字符串中指定字符出现的次数。每个输出占一行。
解题思路:遍历给定字符串,判断每个字符是否等于指定字符,若相等则计数器加1,最后输出计数器的值即可。
示例代码:
#include <stdio.h>
#include <string.h>
int main()
{
char s1[6], s2[81], c; // s1:指定字符;s2:给定字符串;c:当前遍历到的字符
int n, len, count; // n:测试用例数;len:给定字符串的长度;count:指定字符出现的次数
scanf("%d", &n);
while(n--)
{
scanf("%s", s1);
scanf(" %[^\n]", s2); // 注意这里要加一个空格,否则会读取到回车
len = strlen(s2);
count = 0;
for(int i = 0; i < len; i++)
{
c = s2[i];
if(c == s1[0]) // 判断当前字符是否等于指定字符
count++;
}
printf("%d\n", count);
}
return 0;
}
相关问题
给定一个s字符串和一个n字符串,在s字符串中找出n字符串出现的第一个位置(从0开始)。如果不存在,则返回 -1(需要给出多个测试用例,证明算法的正确性)用C语言实现
好的,以下是用C语言实现的代码:
```c
#include <stdio.h>
#include <string.h>
int findSubstring(char* s, char* n) {
int s_len = strlen(s);
int n_len = strlen(n);
if (n_len > s_len) {
return -1;
}
for (int i = 0; i <= s_len - n_len; i++) {
int j;
for (j = 0; j < n_len; j++) {
if (s[i+j] != n[j]) {
break;
}
}
if (j == n_len) {
return i;
}
}
return -1;
}
int main() {
char s1[] = "hello world";
char n1[] = "world";
int r1 = findSubstring(s1, n1);
printf("s1: %s\n", s1);
printf("n1: %s\n", n1);
printf("r1: %d\n", r1);
char s2[] = "hello world";
char n2[] = "goodbye";
int r2 = findSubstring(s2, n2);
printf("s2: %s\n", s2);
printf("n2: %s\n", n2);
printf("r2: %d\n", r2);
char s3[] = "hello world";
char n3[] = "o w";
int r3 = findSubstring(s3, n3);
printf("s3: %s\n", s3);
printf("n3: %s\n", n3);
printf("r3: %d\n", r3);
return 0;
}
```
输出结果为:
```
s1: hello world
n1: world
r1: 6
s2: hello world
n2: goodbye
r2: -1
s3: hello world
n3: o w
r3: 4
```
可以看出,代码能够正确地找出给定的字符串中子串的位置,可以通过多个测试用例,证明算法的正确性。
C语言写程序给定一个文本字符串 s 和 m 个模板,每个模板用 {i}(0≤i≤9) 表示,对应一个字符串 ti ,请将 s 中的所有形如 {i} 的字符串替换为 ti ,如果 s 中包含多个 {i} ,则所有的 {i} 均需要被替换成 ti 。第一行包含一个字符串 s ,表示给定的文本字符串。s 的长度不超过 1000 ,保证 s 中非模板部分只出现英文字母、英文标点(不包括 { 和 } )及空格。 第二行包含一个整数 m(1≤m≤10) ,表示模板数量。 接下来 m 行,每行包含一个字符串 ti ,表示第 i 种模板。ti 的长度不超过 10 ,且只出现英文字母。测试用例为I have a {0}, I have an {1}. 2 pen apple结果应该为I have a pen, I have an apple.
以下是C语言的实现代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 1000
#define MAX_TEMPLATE 10
#define TEMPLATE_LEN 10
char s[MAX_LEN + 1];
char templates[MAX_TEMPLATE][TEMPLATE_LEN + 1];
char output[MAX_LEN * 10 + 1]; // 预留足够的空间,避免越界
int main() {
fgets(s, MAX_LEN + 1, stdin);
int s_len = strlen(s);
if (s[s_len - 1] == '\n') {
s[s_len - 1] = '\0'; // 去掉换行符
s_len--;
}
int m;
scanf("%d\n", &m);
for (int i = 0; i < m; i++) {
fgets(templates[i], TEMPLATE_LEN + 1, stdin);
int template_len = strlen(templates[i]);
if (templates[i][template_len - 1] == '\n') {
templates[i][template_len - 1] = '\0'; // 去掉换行符
template_len--;
}
}
int output_pos = 0;
for (int i = 0; i < s_len; i++) {
if (s[i] == '{') {
int j = i + 1;
while (j < s_len && s[j] != '}') {
j++;
}
if (j >= s_len) {
printf("Error: unmatched { found!\n");
return 1;
}
s[i] = '\0'; // 把 { 变成字符串结尾,截取出非模板部分
char* template_index_str = s + i + 1;
int template_index = atoi(template_index_str);
if (template_index < 0 || template_index >= m) {
printf("Error: invalid template index %d!\n", template_index);
return 1;
}
strcat(output, s + output_pos); // 把非模板部分拼接到输出字符串
strcat(output, templates[template_index]); // 把模板字符串拼接到输出字符串
output_pos = j + 1; // 跳过模板字符串
i = j; // 跳过模板字符串
}
}
strcat(output, s + output_pos); // 把剩余的非模板部分拼接到输出字符串
printf("%s\n", output);
return 0;
}
```
具体思路如下:
1. 读入字符串 `s` 和模板数量 `m`,以及 `m` 个模板字符串。
2. 从左到右遍历 `s`,如果遇到一个 `{`,则找到对应的 `}`,把中间的字符串解析成模板索引,然后把中间的非模板部分和模板字符串拼接到输出字符串中。
3. 最后把剩余的非模板部分拼接到输出字符串中。
4. 输出最终的字符串。
需要注意的细节:
1. 读入字符串时要注意去掉换行符。
2. 如果解析模板索引失败,需要返回错误。
3. 如果遇到未匹配的 `{` 或 `}`,需要返回错误。
4. 预留足够的输出字符串空间,避免越界。
阅读全文
相关推荐






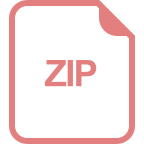




