你好,请基于node和vue写一段,根据文件在服务器的路径下载该文件的后端api接口与相对的前端函数
时间: 2023-09-11 11:05:12 浏览: 121
### 回答1:
前端函数:function downloadFile (url) {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onload = () => {
const {status, response} = xhr;
if (status === 200) {
const fileName = response.split('/').pop();
const blob = new Blob([response], {type: 'application/octet-stream'});
const link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = fileName;
link.click();
}
};
xhr.send();
}后端API接口:app.get('/downloadFile', (req, res) => {
const {filePath} = req.query;
fs.readFile(filePath, (err, data) => {
if (err) {
return res.status(500).send('Error');
}
res.download(filePath);
});
});
### 回答2:
后端API接口:
```javascript
// server.js
const express = require('express');
const app = express();
const path = require('path');
app.get('/download/:filename', (req, res) => {
const filename = req.params.filename;
const filepath = path.join(__dirname, 'files', filename);
res.download(filepath, (err) => {
if (err) {
res.status(404).send('File not found');
}
});
});
app.listen(3000, () => {
console.log('Server started on port 3000');
});
```
前端函数:
```vue
<template>
<div>
<button @click="downloadFile('example.txt')">下载文件</button>
</div>
</template>
<script>
export default {
methods: {
downloadFile(filename) {
fetch(`http://localhost:3000/download/${filename}`)
.then((res) => {
if (!res.ok) {
throw new Error('Request failed');
}
return res.blob();
})
.then((blob) => {
const url = window.URL.createObjectURL(blob);
const a = document.createElement('a');
a.href = url;
a.download = filename;
a.click();
window.URL.revokeObjectURL(url);
})
.catch((error) => {
console.log(error);
});
},
},
};
</script>
```
上面给出了一个简单的基于Node.js和Vue的文件下载示例。首先,在后端代码中,使用Express创建一个服务器,监听在3000端口。当客户端发起/download/:filename的GET请求时,服务器会查找并发送指定文件到客户端。文件路径是根据服务器文件夹路径和传入的文件名拼接而成。如果文件不存在,服务器会返回404错误。
在前端代码中,当用户点击下载按钮时,前端调用`downloadFile`函数,通过fetch发送GET请求到服务器/api/download/:filename路由。如果请求成功,得到一个Blob对象,从中提取文件URL,并创建一个临时a标签,设置下载属性和URL,模拟点击触发文件下载。然后通过revokeObjectURL释放URL对象。
这样,前端用户就能根据文件在服务器的路径下载该文件了。需要注意的是,服务器端需要事先存在待下载的文件,且前后端需运行在同一台设备上。
### 回答3:
后端接口代码:
在Node.js中,我们可以使用`express`框架来搭建后端API。假设我们的后端服务器地址为`http://localhost:3000`,并且有一个文件路径为`/files/download`的接口用于下载文件。
```javascript
const express = require('express');
const app = express();
app.get('/files/download', (req, res) => {
const filePath = req.query.path; // 通过query参数获取文件路径
const fileName = filePath.split('/').pop(); // 获取文件名
// 下载文件
res.download(filePath, fileName, (err) => {
if (err) {
res.status(500).send('下载文件发生错误');
}
});
});
app.listen(3000, () => {
console.log('服务器已启动');
});
```
该接口接受一个名为`path`的query参数,表示要下载的文件在服务器的路径。使用`res.download`方法将文件发送到客户端进行下载。
前端函数代码:
在Vue中,我们可以使用`axios`库来进行前端的HTTP请求。假设我们使用一个名为`downloadFile`的函数来触发文件下载。
```vue
<template>
<button @click="downloadFile">下载文件</button>
</template>
<script>
import axios from 'axios';
export default {
methods: {
downloadFile() {
const filePath = '/path/to/file.txt'; // 文件在服务器的路径
const apiUrl = 'http://localhost:3000/files/download'; // 后端API接口地址
axios.get(apiUrl, {
params: {
path: filePath
},
responseType: 'blob' // 以二进制方式接受响应
})
.then((response) => {
const url = window.URL.createObjectURL(new Blob([response.data]));
const link = document.createElement('a');
link.href = url;
link.setAttribute('download', filePath.split('/').pop());
document.body.appendChild(link);
link.click();
})
.catch((error) => {
console.error('下载文件发生错误', error);
});
}
}
}
</script>
```
这段前端函数首先使用`axios`发送GET请求到后端API接口,并将文件路径作为`path`参数传递。设置`responseType`为`blob`以接受二进制的响应。
在成功获取到响应后,我们使用`Blob`对象创建一个可下载的URL,并使用动态创建的`<a>`元素来触发下载操作。最后,将新创建的`<a>`元素添加到`document.body`中以保证其正常工作,在点击链接后,文件将以指定的文件名下载到本地。
以上就是根据文件在服务器路径下载文件的后端API接口和相对应的前端函数的实现。
阅读全文
相关推荐
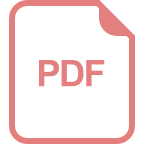
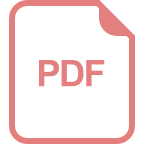
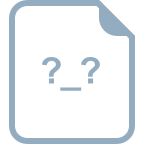
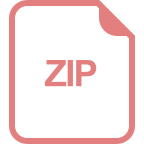
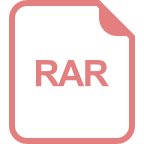
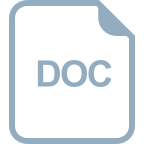
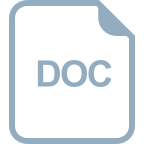
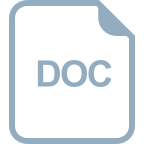
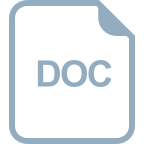
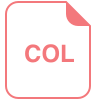
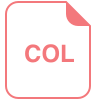
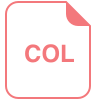
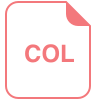
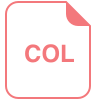
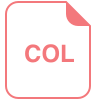
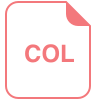
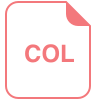