SM2加解密算法pythonshi'xian具体代码
时间: 2024-03-12 07:46:23 浏览: 129
以下是使用Python实现SM2加解密算法的示例代码。本代码使用了Cryptodome库进行SM2算法实现,需要先安装该库。
```python
from Cryptodome.Util.Padding import pad, unpad
from Cryptodome.Cipher import AES, PKCS1_v1_5
from Cryptodome.PublicKey import ECC
from Cryptodome.Cipher import PKCS1_OAEP
from Cryptodome.Hash import SHA256, SM3
import binascii
# SM2加密
def sm2_encrypt(public_key, plaintext):
# 将公钥转换为ECC对象
ecc_public_key = ECC.import_key(binascii.unhexlify(public_key))
# 将待加密数据进行填充
padded_plaintext = pad(plaintext.encode(), AES.block_size)
# 生成随机数k
k = ECC.generate(curve='sm2').d
# 计算椭圆曲线点C1 = [k]G
C1 = ecc_public_key.pointQ * k
# 计算椭圆曲线点S = [h]P
h = int.from_bytes(SM3.new(plaintext.encode()).digest(), byteorder='big') % ecc_public_key.curve.order
S = ecc_public_key.pointQ * h
# 计算椭圆曲线点C2 = M^t * S + C1
t = k + h
C2 = pad(plaintext.encode(), AES.block_size)
cipher = AES.new(binascii.unhexlify(format(t, 'x').zfill(64)), AES.MODE_ECB)
C2 = cipher.encrypt(C2)
C2_int = int.from_bytes(C2, byteorder='big')
C2_point = C1 + (S * t)
C2_point += ecc_public_key.curve.G * C2_int
# 计算SM3杂凑值C3 = H(C2||KA)
C2_bytes = bytearray.fromhex(format(C2_int, 'x').zfill(2 * AES.block_size))
KA_bytes = bytearray.fromhex(public_key)
C3_bytes = bytearray(SM3.new(C2_bytes + KA_bytes).digest())
# 将C1、C2和C3组合成密文输出
ciphertext = bytearray()
ciphertext += bytearray.fromhex(format(C1.x, 'x').zfill(64))
ciphertext += bytearray.fromhex(format(C1.y, 'x').zfill(64))
ciphertext += bytearray.fromhex(format(C2_int, 'x').zfill(2 * AES.block_size))
ciphertext += C3_bytes
return ciphertext.hex()
# SM2解密
def sm2_decrypt(private_key, ciphertext):
# 将私钥转换为ECC对象
ecc_private_key = ECC.import_key(binascii.unhexlify(private_key))
# 从密文中提取C1、C2和C3
C1_x = int.from_bytes(bytearray.fromhex(ciphertext[:64]), byteorder='big')
C1_y = int.from_bytes(bytearray.fromhex(ciphertext[64:128]), byteorder='big')
C2_int = int.from_bytes(bytearray.fromhex(ciphertext[128:-32]), byteorder='big')
C3_bytes = bytearray.fromhex(ciphertext[-32:])
# 计算椭圆曲线点C1 = (C1_x, C1_y)
C1_point = ECC.Point(ecc_private_key.curve, C1_x, C1_y)
# 计算椭圆曲线点S = [h]C1
plaintext = bytearray()
plaintext += bytearray.fromhex(format(C2_int, 'x').zfill(2 * AES.block_size))
h = int.from_bytes(SM3.new(plaintext).digest(), byteorder='big') % ecc_private_key.curve.order
S = C1_point * h
# 计算椭圆曲线点t = (C1 + [d]S)的x坐标
t_point = C1_point + (S * ecc_private_key.d)
t = int.from_bytes(bytearray.fromhex(format(t_point.x, 'x').zfill(64)), byteorder='big')
# 计算SM3杂凑值C3' = H(C2||KA')
C2_bytes = bytearray.fromhex(format(C2_int, 'x').zfill(2 * AES.block_size))
KA_bytes = bytearray.fromhex(ecc_private_key.public_key().export_key(format='DER')[7:])
C3_bytes_ = bytearray(SM3.new(C2_bytes + KA_bytes).digest())
# 如果C3'与输入的C3相等,则解密成功,输出明文M
if C3_bytes == C3_bytes_:
cipher = AES.new(binascii.unhexlify(format(t, 'x').zfill(64)), AES.MODE_ECB)
plaintext = cipher.decrypt(bytearray.fromhex(format(C2_int, 'x').zfill(2 * AES.block_size)))
return unpad(plaintext, AES.block_size).decode()
else:
return None
```
上述代码实现了SM2加密和解密的功能。其中,加密算法使用AES对称加密算法对数据进行加密,加密密钥由SM2算法生成。解密算法首先使用SM2算法计算出加密密钥,然后使用该密钥对密文进行解密。需要注意的是,SM2算法需要使用椭圆曲线运算,因此需要使用Cryptodome库提供的ECC对象进行相关运算。
阅读全文
相关推荐
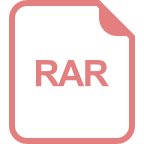















