js java rsa 分段 加解密
时间: 2023-08-05 07:07:38 浏览: 57
RSA加密和解密过程中需要对数据进行分段操作,以保证数据的安全性和加密效率。下面是JS和Java两种语言的RSA分段加解密示例代码:
JS代码:
```javascript
// RSA加密
function rsaEncrypt(text, publicKey) {
const buffer = Buffer.from(text);
const blockSize = publicKey.keySize / 8 - 11; // 1024位密钥,每段最大长度为117
let result = "";
for (let i = 0; i < buffer.length; i += blockSize) {
const chunk = buffer.slice(i, i + blockSize);
const encrypted = publicKey.encrypt(chunk);
result += encrypted.toString("base64");
}
return result;
}
// RSA解密
function rsaDecrypt(text, privateKey) {
const buffer = Buffer.from(text, "base64");
const blockSize = privateKey.keySize / 8; // 1024位密钥,每段最大长度为128
let result = "";
for (let i = 0; i < buffer.length; i += blockSize) {
const chunk = buffer.slice(i, i + blockSize);
const decrypted = privateKey.decrypt(chunk);
result += decrypted.toString();
}
return result;
}
```
Java代码:
```java
// RSA加密
public static String rsaEncrypt(String text, PublicKey publicKey) throws Exception {
byte[] bytes = text.getBytes("UTF-8");
int blockSize = publicKey.getModulus().bitLength() / 8 - 11; // 1024位密钥,每段最大长度为117
ByteArrayOutputStream out = new ByteArrayOutputStream();
int i = 0;
while (i < bytes.length) {
int length = Math.min(blockSize, bytes.length - i);
byte[] chunk = Arrays.copyOfRange(bytes, i, i + length);
byte[] encrypted = rsaEncrypt(chunk, publicKey);
out.write(encrypted);
i += blockSize;
}
return Base64.getEncoder().encodeToString(out.toByteArray());
}
private static byte[] rsaEncrypt(byte[] data, PublicKey publicKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, publicKey);
return cipher.doFinal(data);
}
// RSA解密
public static String rsaDecrypt(String text, PrivateKey privateKey) throws Exception {
byte[] bytes = Base64.getDecoder().decode(text);
int blockSize = privateKey.getModulus().bitLength() / 8; // 1024位密钥,每段最大长度为128
ByteArrayOutputStream out = new ByteArrayOutputStream();
int i = 0;
while (i < bytes.length) {
int length = Math.min(blockSize, bytes.length - i);
byte[] chunk = Arrays.copyOfRange(bytes, i, i + length);
byte[] decrypted = rsaDecrypt(chunk, privateKey);
out.write(decrypted);
i += blockSize;
}
return new String(out.toByteArray(), "UTF-8");
}
private static byte[] rsaDecrypt(byte[] data, PrivateKey privateKey) throws Exception {
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
return cipher.doFinal(data);
}
```
以上代码均是用的是PKCS#1 v1.5填充方式,如果需要使用其他填充方式,需要相应地修改代码。
相关推荐
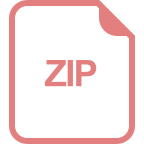
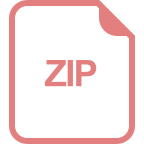
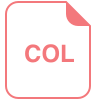













