数据结构栈的实现c++
时间: 2023-05-31 11:06:43 浏览: 150
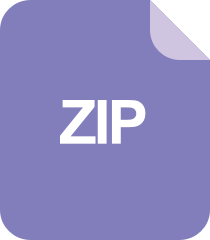
基于C++实现的基础数据结构、泛型数据结构及高阶数据结构,含链式栈、顺序栈、单链表、带头双向循环链表、顺序表、泛型数据结构
以下是一个简单的数据结构栈的实现代码,使用了C语言:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_full(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, int item) {
if (is_full(s)) {
printf("Stack is full\n");
exit(1);
}
s->data[++(s->top)] = item;
}
int pop(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty\n");
exit(1);
}
return s->data[(s->top)--];
}
int peek(Stack *s) {
if (is_empty(s)) {
printf("Stack is empty\n");
exit(1);
}
return s->data[s->top];
}
int main() {
Stack s;
init(&s);
push(&s, 1);
push(&s, 2);
push(&s, 3);
printf("%d\n", peek(&s)); // 3
printf("%d\n", pop(&s)); // 3
printf("%d\n", pop(&s)); // 2
printf("%d\n", pop(&s)); // 1
return 0;
}
```
这个实现使用了数组来存储栈内元素,`top`表示栈顶的位置,初始化为-1。`is_empty`和`is_full`函数分别判断栈是否为空和已满。`push`函数在栈顶添加元素,如果栈已满则会抛出异常。`pop`函数弹出栈顶元素,如果栈已空也会抛出异常。`peek`函数返回栈顶元素,但不弹出。最后在主函数中测试了一下这些函数的使用。
阅读全文
相关推荐
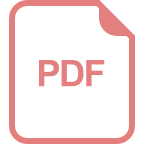
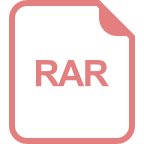
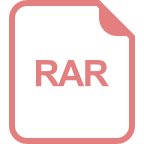
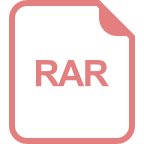
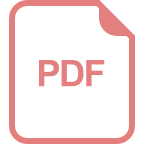
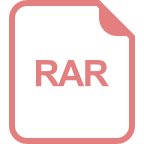
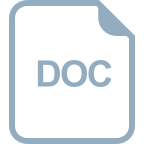
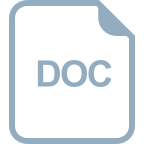
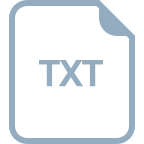
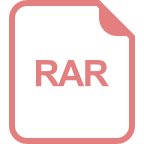
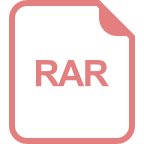
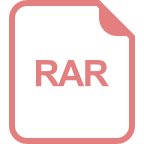
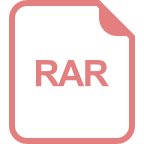