c++ cin读取字符串
时间: 2023-11-27 17:09:42 浏览: 54
在 C++ 中,我们可以使用 `cin` 读取字符串。具体实现方式如下:
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string str;
cout << "请输入一个字符串:";
cin >> str; // 读取字符串
cout << "你输入的字符串是:" << str << endl;
return 0;
}
```
上述代码中,我们通过 `cin` 读取字符串,并把结果存储到 `string` 类型的变量 `str` 中。需要注意的是,`cin` 读取字符串时,会在遇到空格、换行符等空白字符时停止读取,因此如果需要读取带空格的字符串,可以使用 `getline` 函数。
相关问题
cin读取带空格的字符串
cin可以读取带有空格的字符串。在C++中,使用cin来接收用户的输入,可以使用以下方式读取带有空格的字符串。
首先,可以使用getline函数来读取一行带有空格的字符串。getline函数的使用方法如下:
```
string input;
getline(cin, input);
```
上述代码会从标准输入中读取一行字符串,直到遇到换行符为止。这意味着可以读取包含空格的字符串。
另外,也可以使用cin读取字符串,但是需要使用string类的成员函数,例如getline或者ignore。下面是一个使用ignore函数读取带有空格的字符串的例子:
```
string input;
cin >> ws; // 忽略输入流中的前面的空格(ws: whitespace)
getline(cin, input);
```
使用ignore函数可以忽略输入流中的前面的空格,然后使用getline函数读取整行字符串,包括空格。注意,使用cin来读取带有空格的字符串时,空格会被当作分隔符,会自动截断字符串。所以在使用cin读取带有空格的字符串时,需要使用以上两种方法之一来读取整行字符串,以确保可以正确读取到带有空格的字符串。
c++读取字符串遇到空格停止
在C++中,可以使用getline函数读取一行字符串,如果要在遇到空格时停止读取,可以将空格作为分隔符。具体实现可以参考以下示例代码:
```c++
#include<iostream>
#include<string>
using namespace std;
int main()
{
string str;
getline(cin, str, ' '); // 以空格作为分隔符
cout << str << endl;
return 0;
}
```
在上述代码中,使用getline函数读取一行字符串,第二个参数是读取的字符串变量,第三个参数是分隔符,这里使用空格作为分隔符。当读取到空格时,getline函数会停止读取,将之前读取的字符串存储在str变量中。最后输出str变量即可。
相关推荐
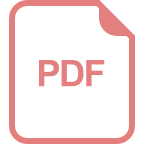
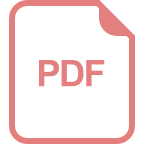
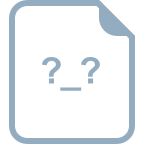












