java 代理模式 例子
时间: 2023-05-20 18:02:09 浏览: 134
一个常见的 Java 代理模式的例子是使用动态代理来实现日志记录。在这种情况下,我们可以创建一个接口,然后使用动态代理来创建一个代理类,该代理类将在调用接口方法之前和之后记录日志。以下是一个简单的示例代码:
```
public interface UserService {
void addUser(String username, String password);
}
public class UserServiceImpl implements UserService {
@Override
public void addUser(String username, String password) {
// 添加用户的具体实现
}
}
public class UserServiceProxy implements InvocationHandler {
private Object target;
public UserServiceProxy(Object target) {
this.target = target;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("Before method " + method.getName());
Object result = method.invoke(target, args);
System.out.println("After method " + method.getName());
return result;
}
}
public class Main {
public static void main(String[] args) {
UserService userService = new UserServiceImpl();
UserService proxy = (UserService) Proxy.newProxyInstance(
userService.getClass().getClassLoader(),
userService.getClass().getInterfaces(),
new UserServiceProxy(userService));
proxy.addUser("test", "123456");
}
}
```
在这个例子中,我们定义了一个 UserService 接口和它的实现类 UserServiceImpl。然后,我们创建了一个 UserServiceProxy 类,它实现了 InvocationHandler 接口,并在 invoke 方法中记录了日志。最后,我们在 Main 类中使用动态代理来创建一个代理对象,并调用 addUser 方法。当 addUser 方法被调用时,代理对象将记录日志并调用实际的 addUser 方法。
阅读全文
相关推荐




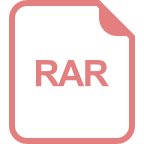

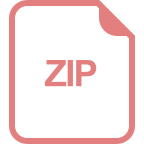





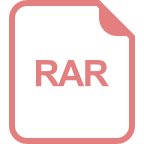




