帮我根据以下要求:Add to the Model class a new method called savaData that saves into a text file called "points.txt" the integer coordinates x and y of each point in the arraylist of points. Also modify the constructor of the Model class to read the integer coordinates of all the points from the same text file, if it exists, and put them into the arraylist of points (if the file does not exist then the arraylist of points should remain empty).public class Model { private ArrayList<Point> points; private ArrayList<ModelListener> listeners; public Model() { points = new ArrayList<Point>(); listeners = new ArrayList<ModelListener>(); } public void addListener(ModelListener l) { listeners.add(l); } public ArrayList<Point> getPoints() { return points; } public void addPoint(Point p) { points.add(p); notifyListeners(); // points changed so notify the listeners. } public void clearAllPoints() { points.clear(); notifyListeners(); // points changed so notify the listeners. } public void deleteLastPoint() { if(points.size() > 0) { points.remove(points.size() - 1); notifyListeners(); // points changed so notify the listeners. } } private void notifyListeners() { for(ModelListener l: listeners) { l.update(); // Tell the listener that something changed. } } public int numberOfPoints() { return points.size(); } public static void testModel() { Model m = new Model(); m.addListener(new ModelListener() { @Override public void update() { System.out.println(true + " (listener)"); } }); System.out.println(m.getPoints() == m.points); Point p1 = new Point(1, 2); Point p2 = new Point(3, 4); m.addPoint(p1); // Listener called. m.addPoint(p2); // Listener called. System.out.println(m.numberOfPoints() == 2); System.out.println(m.points.get(0) == p1); System.out.println(m.points.get(1) == p2); m.deleteLastPoint(); // Listener called. System.out.println(m.numberOfPoints() == 1); System.out.println(m.points.get(0) == p1); m.clearAllPoints(); // Listener called. System.out.println(m.numberOfPoints() == 0); m.notifyListeners(); // Listener called. } }修改下述代码:
时间: 2024-01-07 17:06:12 浏览: 57
public class Model {
private ArrayList<Point> points;
private ArrayList<ModelListener> listeners;
public Model() {
points = new ArrayList<Point>();
listeners = new ArrayList<ModelListener>();
// Read points from file if it exists
File file = new File("points.txt");
if (file.exists()) {
try {
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String[] coordinates = scanner.nextLine().split(" ");
int x = Integer.parseInt(coordinates[0]);
int y = Integer.parseInt(coordinates[1]);
points.add(new Point(x, y));
}
scanner.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
public void addListener(ModelListener l) {
listeners.add(l);
}
public ArrayList<Point> getPoints() {
return points;
}
public void addPoint(Point p) {
points.add(p);
notifyListeners(); // points changed so notify the listeners.
saveData(); // save point to file
}
public void clearAllPoints() {
points.clear();
notifyListeners(); // points changed so notify the listeners.
saveData(); // save empty list to file
}
public void deleteLastPoint() {
if(points.size() > 0) {
points.remove(points.size() - 1);
notifyListeners(); // points changed so notify the listeners.
saveData(); // save updated list to file
}
}
private void notifyListeners() {
for(ModelListener l: listeners) {
l.update(); // Tell the listener that something changed.
}
}
public int numberOfPoints() {
return points.size();
}
public void saveData() {
try {
FileWriter writer = new FileWriter("points.txt");
for (Point p : points) {
writer.write(p.x + " " + p.y + "\n");
}
writer.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void testModel() {
Model m = new Model();
m.addListener(new ModelListener() {
@Override
public void update() {
System.out.println(true + " (listener)");
}
});
System.out.println(m.getPoints() == m.points);
Point p1 = new Point(1, 2);
Point p2 = new Point(3, 4);
m.addPoint(p1); // Listener called.
m.addPoint(p2); // Listener called.
System.out.println(m.numberOfPoints() == 2);
System.out.println(m.points.get(0) == p1);
System.out.println(m.points.get(1) == p2);
m.deleteLastPoint(); // Listener called.
System.out.println(m.numberOfPoints() == 1);
System.out.println(m.points.get(0) == p1);
m.clearAllPoints(); // Listener called.
System.out.println(m.numberOfPoints() == 0);
m.notifyListeners(); // Listener called.
}
}
阅读全文
相关推荐
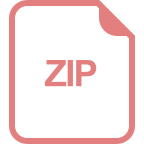










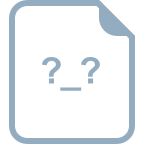
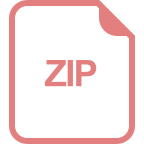
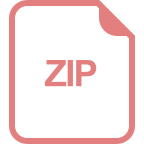
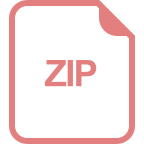
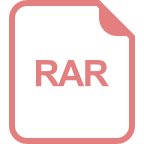
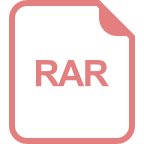
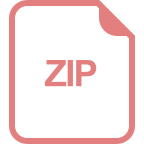