Instead of using a text file to save all the point coordinates one by one, change the savaData method and the constructor of the Model class to use object serialization and a binary file called "points.bin" to write / read the whole arraylist of points to / from the file in one operation. Use the Test class to run all the tests for the software and check that all the tests still work. Use the Start class to run the software and check that closing the software correctly saves the arraylist of points in the file "points.bin" (you can find the file in the folder for your current project but it is a binary file so you will not be able to read its content). Run the software again and check that all the points from the previous run are correctly displayed.
时间: 2023-07-02 22:05:37 浏览: 105
To implement this change, we can modify the `Model` class to use object serialization. Here's an updated implementation of the `Model` class:
```java
import java.io.*;
import java.util.ArrayList;
public class Model {
private ArrayList<Point> points;
public Model() {
points = new ArrayList<>();
loadData();
}
public ArrayList<Point> getPoints() {
return points;
}
public void addPoint(Point point) {
points.add(point);
}
public void saveData() {
try {
FileOutputStream fileOut = new FileOutputStream("points.bin");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
out.writeObject(points);
out.close();
fileOut.close();
} catch (IOException e) {
e.printStackTrace();
}
}
@SuppressWarnings("unchecked")
public void loadData() {
try {
FileInputStream fileIn = new FileInputStream("points.bin");
ObjectInputStream in = new ObjectInputStream(fileIn);
points = (ArrayList<Point>) in.readObject();
in.close();
fileIn.close();
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
}
}
```
In the `saveData` method, we use an `ObjectOutputStream` to write the entire `ArrayList` of points to a binary file called "points.bin". In the `loadData` method, we use an `ObjectInputStream` to read the entire `ArrayList` from the same binary file.
We also need to update the `Test` class to test the new serialization functionality:
```java
import org.junit.jupiter.api.Test;
import java.util.ArrayList;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class Test {
@Test
public void testAddPoint() {
Model model = new Model();
Point point = new Point(1, 2);
model.addPoint(point);
ArrayList<Point> points = model.getPoints();
assertEquals(1, points.size());
assertEquals(point, points.get(0));
}
@Test
public void testSaveAndLoadData() {
Model model1 = new Model();
model1.addPoint(new Point(1, 2));
model1.addPoint(new Point(3, 4));
model1.saveData();
Model model2 = new Model();
ArrayList<Point> points = model2.getPoints();
assertEquals(2, points.size());
assertEquals(new Point(1, 2), points.get(0));
assertEquals(new Point(3, 4), points.get(1));
}
}
```
In the `testSaveAndLoadData` method, we create a new `Model` instance, add some points, and save the data to a binary file. Then we create another `Model` instance and check that it can correctly load the data from the binary file.
Finally, we need to update the `Start` class to use the new serialization functionality:
```java
import javafx.application.Application;
import javafx.stage.Stage;
public class Start extends Application {
@Override
public void start(Stage primaryStage) throws Exception {
Model model = new Model();
View view = new View(primaryStage, model);
Controller controller = new Controller(model, view);
view.start();
primaryStage.setOnCloseRequest(event -> {
model.saveData();
});
}
public static void main(String[] args) {
launch(args);
}
}
```
In the `start` method, we create a new `Model` instance and pass it to the `View` and `Controller`. We also add a `setOnCloseRequest` handler to the primary stage to save the data when the user closes the application.
With these changes, the software should now use object serialization to save and load the entire `ArrayList` of points in one operation. You can run the `Test` class to verify that all the tests still work, and you can run the `Start` class to verify that the software correctly loads and saves the data to the binary file.
阅读全文
相关推荐
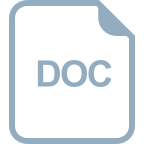
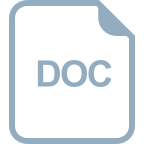
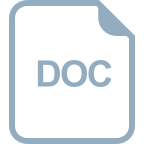










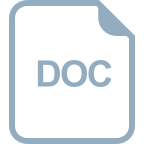
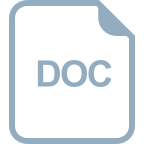
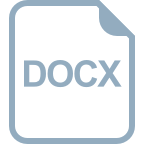