帮我写出以下java代码:The clearInvisibles method takes as argument the width w and the height h of the window, and deletes from the arraylist of bubbles any bubble which is not visible in the window anymore. For each bubble which is deleted, the score decreases by 1. WARNING: when you use the remove method of Java’s ArrayList class to remove an element of an arraylist at index i, the arraylist immediately shifts down by one position all the elements with higher indexes to make the arraylist one element shorter. So, for example, when removing the element at index i, the element at index i+1 immediately moves to the position at index i, the element at index i+2 immediately moves to the position at index i+1, etc. This means that on the next iteration of the loop, when i has become i+1, the element that you will be testing at index i+1 is in fact the element that used to be at index i+2. Which means that the element that used to be at index i+1 (and which is now at index i) will never be tested! Therefore, when removing elements from an arraylist, if your loop starts at index 0 and goes up the indexes in the arraylist, then your loop will fail to test some elements! CONCLUSION: when removing elements from an arraylist, your loop must start from the END of the arraylist and go DOWN to index 0. The deleteBubblesAtPoint method takes as argument the coordinates (x, y) of a point, and deletes from the arraylist of bubbles any bubble which contains this point (multiple bubbles might contain the point, because bubbles can overlap in the window). For each bubble which is deleted, the score increases by 1. The drawAll method draws all the bubbles in the arraylist of bubbles. Make sure you test as many methods of the Model class as poss
时间: 2023-06-27 20:04:16 浏览: 82
public class Model {
private ArrayList<Bubble> bubbles;
private int score;
public void clearInvisibles(int w, int h) {
for (int i = bubbles.size() - 1; i >= 0; i--) {
Bubble bubble = bubbles.get(i);
if (!bubble.isVisible(w, h)) {
bubbles.remove(i);
score--;
}
}
}
public void deleteBubblesAtPoint(int x, int y) {
Iterator<Bubble> iterator = bubbles.iterator();
while (iterator.hasNext()) {
Bubble bubble = iterator.next();
if (bubble.containsPoint(x, y)) {
iterator.remove();
score++;
}
}
}
public void drawAll() {
for (Bubble bubble : bubbles) {
bubble.draw();
}
}
}
class Bubble {
private int x;
private int y;
private int radius;
public boolean isVisible(int w, int h) {
return (x + radius >= 0 && x - radius <= w && y + radius >= 0 && y - radius <= h);
}
public boolean containsPoint(int x, int y) {
return (Math.pow((this.x - x), 2) + Math.pow((this.y - y), 2) <= Math.pow(radius, 2));
}
public void draw() {
// code to draw bubble
}
}
阅读全文
相关推荐
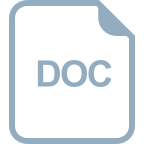
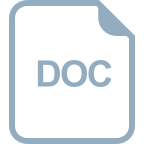
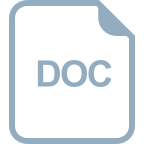















