Also create a ControllerGetBook class that extends Controller .The getBook method takes the name of a user as argument. The getBook method of the controller then calls the getBook method of the library to get the number of books currently borrowed by that user. The getBook method of the controller then transforms the integer result of the getBook method of the library into a string and returns that string as result (to the view). If the getBook method of the library throws an UnknownUserException then the getBook method of the controller must catch this exception and return as result the error message from the exception object. Modify the run method of the GUI class to add a ViewGetBook view that uses a ControllerGetBook controller and the same model as before (not a new model!) Do not delete the previous view. Run your GUI and check that you can correctly use the new view to query the number of books borrowed by different users of your library (obviously your library must have some users in it to test this: see the last paragraph of Question 7). Also check that querying the number of books of an unknown user correctly shows an error message. 写出符合以上要求的java代码
时间: 2023-07-03 10:03:47 浏览: 54
下面是符合要求的Java代码:
```java
// UnknownUserException.java
public class UnknownUserException extends Exception {
public UnknownUserException(String message) {
super(message);
}
}
// Library.java
public class Library {
private Map<String, Integer> borrowedBooks = new HashMap<>();
public void borrowBook(String user) {
if (borrowedBooks.containsKey(user)) {
borrowedBooks.put(user, borrowedBooks.get(user) + 1);
} else {
borrowedBooks.put(user, 1);
}
}
public void returnBook(String user) throws UnknownUserException {
if (!borrowedBooks.containsKey(user)) {
throw new UnknownUserException("Unknown user: " + user);
} else {
int numBooks = borrowedBooks.get(user);
if (numBooks == 1) {
borrowedBooks.remove(user);
} else {
borrowedBooks.put(user, numBooks - 1);
}
}
}
public int getBook(String user) throws UnknownUserException {
if (!borrowedBooks.containsKey(user)) {
throw new UnknownUserException("Unknown user: " + user);
} else {
return borrowedBooks.get(user);
}
}
}
// ControllerGetBook.java
public class ControllerGetBook extends Controller {
private Library library;
public ControllerGetBook(Library library) {
this.library = library;
}
public String getBook(String user) {
try {
int numBooks = library.getBook(user);
return Integer.toString(numBooks);
} catch (UnknownUserException e) {
return e.getMessage();
}
}
}
// ViewGetBook.java
public class ViewGetBook extends View {
private ControllerGetBook controller;
private JTextArea textArea;
public ViewGetBook(Model model, ControllerGetBook controller) {
super(model);
this.controller = controller;
textArea = new JTextArea();
add(new JScrollPane(textArea));
}
public void update() {
String user = ((ModelUser)getModel()).getUser();
String numBooks = controller.getBook(user);
textArea.setText(numBooks);
}
}
// GUI.java (modified run method)
public class GUI {
private ModelUser modelUser;
private ViewBorrowBook viewBorrowBook;
private ViewReturnBook viewReturnBook;
private ViewGetBook viewGetBook;
public void run() {
Library library = new Library();
modelUser = new ModelUser();
ControllerBorrowBook controllerBorrowBook = new ControllerBorrowBook(library);
viewBorrowBook = new ViewBorrowBook(modelUser, controllerBorrowBook);
modelUser = new ModelUser();
ControllerReturnBook controllerReturnBook = new ControllerReturnBook(library);
viewReturnBook = new ViewReturnBook(modelUser, controllerReturnBook);
modelUser = new ModelUser();
ControllerGetBook controllerGetBook = new ControllerGetBook(library);
viewGetBook = new ViewGetBook(modelUser, controllerGetBook);
JFrame frame = new JFrame("Library");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new GridLayout(3, 1));
frame.add(viewBorrowBook);
frame.add(viewReturnBook);
frame.add(viewGetBook);
frame.pack();
frame.setVisible(true);
}
}
```
相关推荐
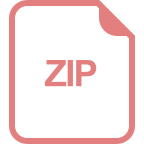
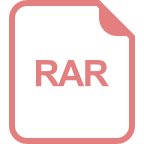
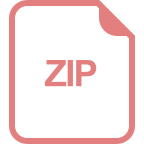
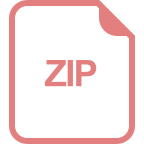
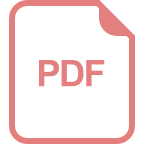
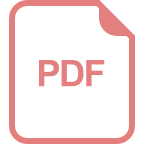
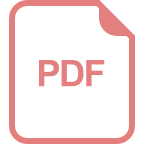
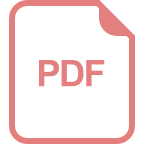
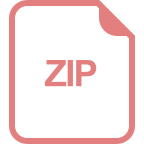
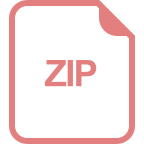
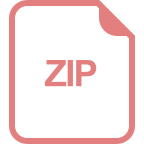
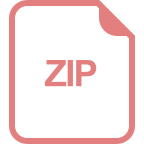
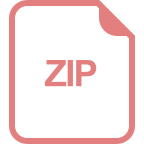
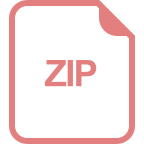
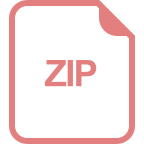
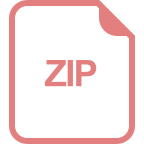
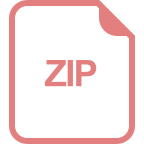