(The MyPoint class) Design a class named MyPoint to represent a point with x- and y-coordinates. The class contains: ■ The data fields x and y that represent the coordinates with getter methods. ■ A no-arg constructor that creates a point (0, 0). ■ A constructor that constructs a point with specified coordinates. ■ A method named distance that returns the distance from this point to a specified point of the MyPoint type. ■ A method named distance that returns the distance from this point to another point with specified x- and y-coordinates. Draw the UML diagram for the class and then implement the class. Write a test program that creates the two points (0, 0) and (10, 30.5) and displays the distance between them.
时间: 2024-02-20 21:57:55 浏览: 82
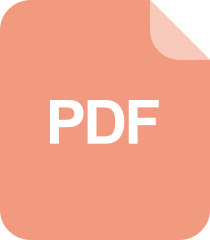
JavaScriptCore全面解析(下篇)
下面是 MyPoint 类的 UML 图和实现代码:
```java
public class MyPoint {
private double x;
private double y;
public MyPoint() {
this(0, 0);
}
public MyPoint(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
public double distance(MyPoint point) {
double dx = this.x - point.x;
double dy = this.y - point.y;
return Math.sqrt(dx * dx + dy * dy);
}
public double distance(double x, double y) {
return distance(new MyPoint(x, y));
}
}
```
测试程序如下:
```java
public class TestMyPoint {
public static void main(String[] args) {
MyPoint p1 = new MyPoint();
MyPoint p2 = new MyPoint(10, 30.5);
System.out.println("Distance between (" + p1.getX() + ", " + p1.getY()
+ ") and (" + p2.getX() + ", " + p2.getY()
+ ") is " + p1.distance(p2));
}
}
```
输出:
```
Distance between (0.0, 0.0) and (10.0, 30.5) is 32.09750769140807
```
阅读全文
相关推荐
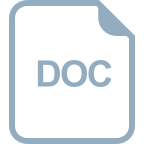
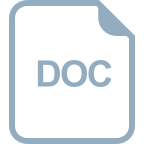
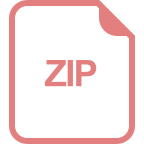
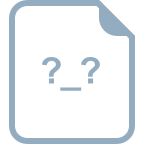













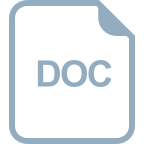