代码We now want to always redraw all the points that have ever been drawn in the panel, not just the last point. To do this, we must save the coordinates of all these points so that we can redraw them all one by one in the paintComponent method every time this method is called. To save the coordinates of the various mouse positions we click, replace the x and y instance variables of the MyPanel class with a single private instance variable called points of type ArrayList<Point>. The Point class is provided to you by Swing. In the constructor of MyPanel, initialize the points instance variable with a new arraylist object of the same type. In the mouseClicked method of the mouse listener, use the getPoint method of the mouse event object to get a Point object representing the position of the mouse click (that Point object internally stores both the x and y coordinates of the mouse click event). Then add this Point object to the arraylist using the arraylist’s add method. Then, in the paintComponent method, add a loop to draw in the panel all the points of the arraylist. You can get the number of elements in the arraylist by using the size method of the arraylist; you can access a specific element of the arraylist at index i by using the get(i) method of the arraylist (element indexes start at zero in an arraylist). The Point class has getX and getY methods to get the coordinates of the point (these two methods return values of type double so you need to cast the returned values into the int type before you can use them to draw a point).
时间: 2023-06-10 09:06:16 浏览: 103
下面是修改后的代码:
```java
import java.awt.*;
import java.awt.event.*;
import java.util.ArrayList;
import javax.swing.*;
public class MyPanel extends JPanel implements MouseListener {
private ArrayList<Point> points;
public MyPanel() {
points = new ArrayList<Point>();
addMouseListener(this);
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
for (int i = 0; i < points.size(); i++) {
Point p = points.get(i);
int x = (int) p.getX();
int y = (int) p.getY();
g.fillOval(x, y, 5, 5);
}
}
public void mouseClicked(MouseEvent e) {
Point p = e.getPoint();
points.add(p);
repaint();
}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
public void mousePressed(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
}
```
现在,我们使用 ArrayList<Point> 类型的 points 变量存储所有点的坐标。在 MyPanel 构造函数中,我们使用 `new ArrayList<Point>()` 初始化 points。在 mouseClicked 方法中,我们使用 `e.getPoint()` 获取点击位置的坐标,然后将其添加到 points 中。在 paintComponent 方法中,我们使用 for 循环遍历 points 中的所有点,并在面板上画出它们。由于 Point 类的 getX 和 getY 方法返回 double 类型的值,我们需要将其转换为 int 类型的值才能使用它们来画一个点。最后,我们调用 `repaint()` 方法来重新绘制面板。
阅读全文
相关推荐
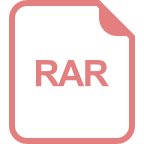
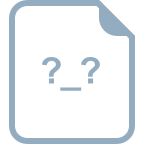
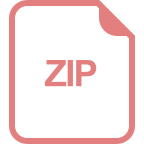
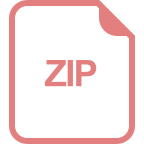
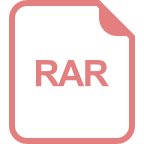
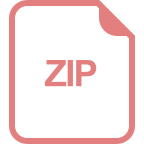
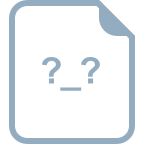
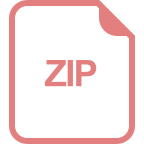










