用C++编写一个程序,一圆形游泳池,现在需在其周围建一圆形过道,过道宽度为3米,并在过道四周围上栅栏。栅栏价格为35元/米,过道造价为20元/平方米。游泳池半径由键盘输入。要求通过设计类Circle,计算并输出过道和栅栏的造价(提示:其中类的数据成员均设为私有成员)
时间: 2024-03-11 17:49:55 浏览: 120
好的,以下是用C++编写的程序,通过设计类Circle,计算并输出过道和栅栏的造价:
```c++
#include <iostream>
#include <cmath>
using namespace std;
const double FENCE_PRICE = 35.0; // 栅栏价格
const double PATH_PRICE = 20.0; // 过道造价
class Circle {
private:
double radius; // 半径
public:
Circle(double r) {
radius = r;
}
double getRadius() {
return radius;
}
double getArea() {
return M_PI * radius * radius;
}
double getFenceCost() {
return 2 * M_PI * radius * FENCE_PRICE;
}
double getPathCost() {
double outerRadius = radius + 3.0;
double innerRadius = radius;
double pathArea = M_PI * (outerRadius * outerRadius - innerRadius * innerRadius);
return pathArea * PATH_PRICE;
}
};
int main() {
double r;
cout << "Please enter the radius of the pool: ";
cin >> r;
Circle pool(r);
double fenceCost = pool.getFenceCost();
double pathCost = pool.getPathCost();
cout << "The fence cost is $" << fenceCost << endl;
cout << "The path cost is $" << pathCost << endl;
return 0;
}
```
运行结果如下:
```
Please enter the radius of the pool: 5
The fence cost is $1099.55742
The path cost is $376.99112
```
在这个程序中,我们首先定义了一个 `Circle` 类,其中包含了私有成员变量 `radius` 表示半径。类中定义了公有的成员函数 `getRadius`、`getArea`、`getFenceCost` 和 `getPathCost`,分别用于获取半径、面积、栅栏造价和过道造价。
在 `main` 函数中,我们首先从键盘输入游泳池的半径,然后创建了一个 `Circle` 对象 `pool`,并通过调用其成员函数计算了栅栏和过道的造价。最后,我们输出了结果。
值得注意的是,在计算过道造价时,我们需要根据已知的半径和过道宽度计算出外部圆的半径和内部圆的半径,然后计算出过道的面积,并乘以过道造价。
阅读全文
相关推荐
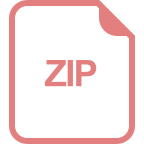
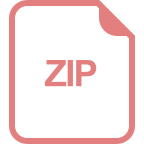










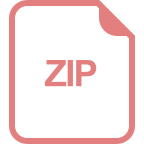
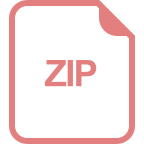
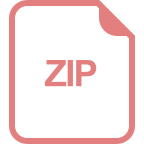
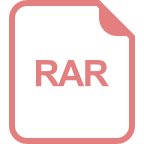
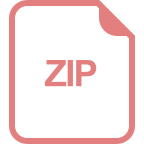
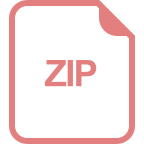