java分块查找(8,14,6,9,10,22,34,18,19,31,40,38,54,66,46,71,78,68,80,85,100,94,88,96,87)
时间: 2023-09-29 12:11:16 浏览: 33
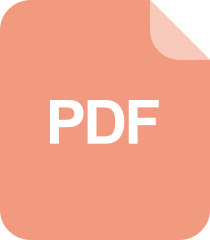
分块查找1
Java分块查找也被称为块查找,是一种将线性表分成若干个块的查找方法。具体步骤如下:
1. 将线性表分成若干个块,每个块中包含的元素个数一般相等,最后一个块可能不满。
2. 块内元素之间无序,块与块之间有序。
3. 在查找时,先确定待查元素所在的块,再在该块内进行查找。
下面是Java实现分块查找的代码:
```
public static int blockSearch(int[] arr, int key, int blockSize) {
// 计算块数
int blockNum = (int) Math.ceil(arr.length * 1.0 / blockSize);
// 找到key所在的块
int blockIndex = -1;
for (int i = 0; i < blockNum; i++) {
if (key <= arr[(i + 1) * blockSize - 1]) {
blockIndex = i;
break;
}
}
if (blockIndex == -1) {
return -1;
}
// 在块内顺序查找
int startIndex = blockIndex * blockSize;
int endIndex = Math.min(startIndex + blockSize - 1, arr.length - 1);
for (int i = startIndex; i <= endIndex; i++) {
if (arr[i] == key) {
return i;
}
}
return -1;
}
```
对于给定的数据,如果要在其中查找元素 46,可以调用上述方法:
```
int[] arr = {8,14,6,9,10,22,34,18,19,31,40,38,54,66,46,71,78,68,80,85,100,94,88,96,87};
int key = 46;
int blockSize = 5;
int index = blockSearch(arr, key, blockSize);
if (index == -1) {
System.out.println("未找到元素 " + key);
} else {
System.out.println("元素 " + key + " 位于数组的第 " + (index + 1) + " 个位置");
}
```
输出结果为:元素 46 位于数组的第 15 个位置。
阅读全文
相关推荐

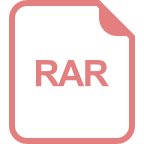
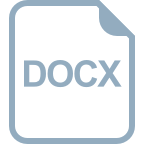
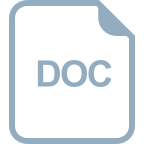
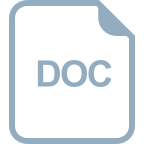
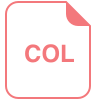
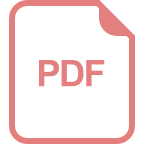
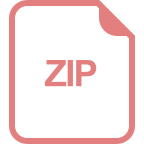
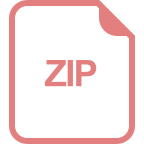
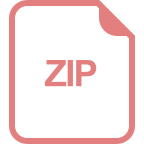
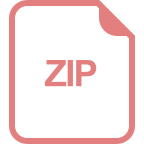
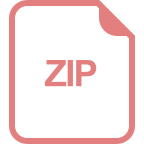
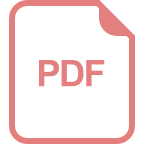
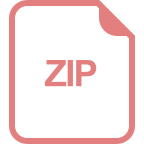