请用python的remi写一个登录注册网页
时间: 2023-12-15 20:06:10 浏览: 48
很抱歉,作为AI语言模型,我无法在本地环境中使用Remi库,因此无法为您提供完整的代码示例。但是,我可以为您提供Remi库的简介和一些代码片段,以帮助您开始编写登录注册网页。
Remi是一个用于创建基于web的用户界面的Python库,它使用Python编写,允许您创建窗口、按钮、文本框等元素,并将它们组合成交互式用户界面。它还提供了一些预定义的组件,例如表格、图表和菜单栏。
下面是一些示例代码片段,可以帮助您开始编写登录和注册页面:
```python
import remi.gui as gui
from remi import start, App
class Login(App):
def __init__(self, *args):
super(Login, self).__init__(*args)
# 创建登录表单元素
self.username = gui.TextInput(width=200, height=30)
self.password = gui.TextInput(width=200, height=30, password=True)
self.login_button = gui.Button('登录', width=200, height=30)
# 将表单元素添加到界面
self.main_container = gui.VBox()
self.main_container.append(gui.Label('用户名'))
self.main_container.append(self.username)
self.main_container.append(gui.Label('密码'))
self.main_container.append(self.password)
self.main_container.append(self.login_button)
# 绑定按钮点击事件
self.login_button.onclick.do(self.login)
# 设置界面
self.main_container.set_style({'margin': 'auto', 'padding': '50px', 'text-align': 'center'})
self.username.set_style({'margin': 'auto', 'text-align': 'center'})
self.password.set_style({'margin': 'auto', 'text-align': 'center'})
self.login_button.set_style({'margin': 'auto'})
# 显示界面
self.main_container.attributes['title'] = '登录'
self.main_container.style['width'] = '300px'
self.main_container.style['height'] = '200px'
self.main_container.style['border'] = '1px solid #ccc'
self.main_container.style['border-radius'] = '5px'
self.main_container.style['background-color'] = '#f9f9f9'
self.main_container.style['box-shadow'] = '0px 0px 10px 0px rgba(0,0,0,0.75)'
self.main_container.style['-webkit-box-shadow'] = '0px 0px 10px 0px rgba(0,0,0,0.75)'
self.main_container.style['-moz-box-shadow'] = '0px 0px 10px 0px rgba(0,0,0,0.75)'
self.main_container.style['position'] = 'absolute'
self.main_container.style['left'] = '50%'
self.main_container.style['top'] = '50%'
self.main_container.style['transform'] = 'translate(-50%, -50%)'
self.main_container.style['z-index'] = '9999'
self.main_container.style['font-family'] = 'Arial'
self.main_container.style['font-size'] = '16px'
self.main_container.style['color'] = '#333'
self.main_container.style['overflow'] = 'hidden'
self.main_container.style['display'] = 'none'
self.append(self.main_container)
def login(self, widget):
# 处理登录逻辑
pass
if __name__ == '__main__':
start(Login)
```
上面的代码创建了一个简单的登录表单,包括用户名、密码和登录按钮。它还定义了一个`login`方法,该方法将在用户单击登录按钮时调用。您需要在该方法中添加处理登录逻辑的代码。
类似地,您可以使用以下代码创建一个简单的注册表单:
```python
class Register(App):
def __init__(self, *args):
super(Register, self).__init__(*args)
# 创建注册表单元素
self.username = gui.TextInput(width=200, height=30)
self.password = gui.TextInput(width=200, height=30, password=True)
self.confirm_password = gui.TextInput(width=200, height=30, password=True)
self.register_button = gui.Button('注册', width=200, height=30)
# 将表单元素添加到界面
self.main_container = gui.VBox()
self.main_container.append(gui.Label('用户名'))
self.main_container.append(self.username)
self.main_container.append(gui.Label('密码'))
self.main_container.append(self.password)
self.main_container.append(gui.Label('确认密码'))
self.main_container.append(self.confirm_password)
self.main_container.append(self.register_button)
# 绑定按钮点击事件
self.register_button.onclick.do(self.register)
# 设置界面
self.main_container.set_style({'margin': 'auto', 'padding': '50px', 'text-align': 'center'})
self.username.set_style({'margin': 'auto', 'text-align': 'center'})
self.password.set_style({'margin': 'auto', 'text-align': 'center'})
self.confirm_password.set_style({'margin': 'auto', 'text-align': 'center'})
self.register_button.set_style({'margin': 'auto'})
# 显示界面
self.main_container.attributes['title'] = '注册'
self.main_container.style['width'] = '300px'
self.main_container.style['height'] = '250px'
self.main_container.style['border'] = '1px solid #ccc'
self.main_container.style['border-radius'] = '5px'
self.main_container.style['background-color'] = '#f9f9f9'
self.main_container.style['box-shadow'] = '0px 0px 10px 0px rgba(0,0,0,0.75)'
self.main_container.style['-webkit-box-shadow'] = '0px 0px 10px 0px rgba(0,0,0,0.75)'
self.main_container.style['-moz-box-shadow'] = '0px 0px 10px 0px rgba(0,0,0,0.75)'
self.main_container.style['position'] = 'absolute'
self.main_container.style['left'] = '50%'
self.main_container.style['top'] = '50%'
self.main_container.style['transform'] = 'translate(-50%, -50%)'
self.main_container.style['z-index'] = '9999'
self.main_container.style['font-family'] = 'Arial'
self.main_container.style['font-size'] = '16px'
self.main_container.style['color'] = '#333'
self.main_container.style['overflow'] = 'hidden'
self.main_container.style['display'] = 'none'
self.append(self.main_container)
def register(self, widget):
# 处理注册逻辑
pass
if __name__ == '__main__':
start(Register)
```
这两个表单可以作为您的登录注册页面的基础,并且可以根据您的需要进行修改和扩展。希望这些示例代码可以帮助您入门Remi库并开始编写自己的登录注册网页!
相关推荐
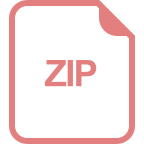
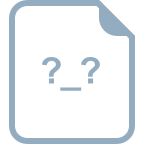
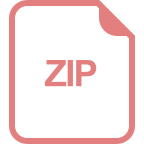














