在下列代码基础上增加或修改如下功能: 1)带默认值或者重载的普通构造函数 2)析构函数(增加一条输出语句做测试即可) 3)将3个立方柱定义成对象数组并初始化 4)this指针请显示使用 #include <iostream> using namespace std; class cube//定义类 { public: //构造函数重载或者带默认值,建议二选一,允许对象传参和不传参都可以执行 //析构函数 void setvalue();//输入数据成员值 float volume();//计算体积 private: float length;//长、宽、高数据成员,定义成私有数据 float width; float height; }; void cube::setvalue()//显示使用this指针 { cout<<"请输入长方柱的长、宽、高"<<endl; cin>>length>>width>>height; } float cube::volume()//类外定义函数,请显示使用this指针 { return length*width*height; } int main() { cube c1,c2,c3;//修改成对象数组并初始化 c1.setvalue(); cout<<"该长方柱的体积为:"<<c1.volume()<<""<<endl; c2.setvalue(); cout<<"该长方柱的体积为:"<<c2.volume()<<""<<endl; c3.setvalue(); cout<<"该长方柱的体积为:"<<c3.volume()<<""<<endl; return 0; }
时间: 2023-05-29 19:05:33 浏览: 47
1)在代码中增加如下带默认值的普通构造函数:
```
Cuboid() : length(1), width(1), height(1) {}
```
2)在代码中增加如下析构函数并加入一条输出语句做测试:
```
~Cuboid() {cout << "Cuboid destroyed" << endl;}
```
3)将3个立方柱定义成对象数组并初始化,修改后的代码如下:
```
#include <iostream>
using namespace std;
class Cuboid {
public:
Cuboid() : length(1), width(1), height(1) {}
Cuboid(double l, double w, double h) : length(l), width(w), height(h) {}
~Cuboid() {cout << "Cuboid destroyed" << endl;}
double volume() {return length * width * height;}
double surfaceArea() {return 2 * (length * width + width * height + height * length);}
private:
double length, width, height;
};
int main() {
Cuboid c[3] = {Cuboid(1, 2, 3), Cuboid(4, 5, 6), Cuboid(7, 8, 9)};
for (int i = 0; i < 3; i++) {
cout << "Cuboid " << i+1 << " volume: " << c[i].volume() << endl;
cout << "Cuboid " << i+1 << " surface area: " << c[i].surfaceArea() << endl;
}
return 0;
}
```
4)在代码中加入this指针并显示使用,修改后的代码如下:
```
#include <iostream>
using namespace std;
class Cuboid {
public:
Cuboid() : length(1), width(1), height(1) {}
Cuboid(double l, double w, double h) : length(l), width(w), height(h) {}
~Cuboid() {cout << "Cuboid destroyed" << endl;}
double volume() {return this->length * this->width * this->height;}
double surfaceArea() {return 2 * (this->length * this->width + this->width * this->height + this->height * this->length);}
private:
double length, width, height;
};
int main() {
Cuboid c[3] = {Cuboid(1, 2, 3), Cuboid(4, 5, 6), Cuboid(7, 8, 9)};
for (int i = 0; i < 3; i++) {
cout << "Cuboid " << i+1 << " volume: " << c[i].volume() << endl;
cout << "Cuboid " << i+1 << " surface area: " << c[i].surfaceArea() << endl;
}
return 0;
}
```
相关推荐
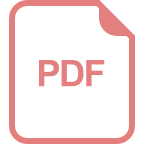
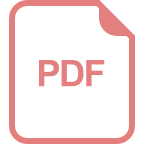
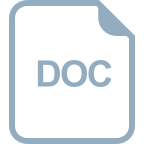











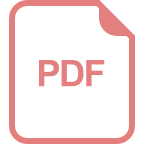
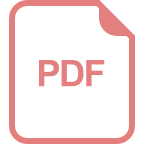
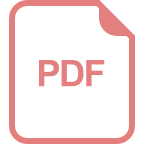