OC中 实现AVPlayer音频下载
时间: 2024-06-08 20:11:08 浏览: 323
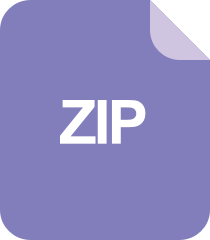
AVPlayer实现的TableViewCell上播放

在 iOS 开发中,可以使用 AVPlayer 来播放音频,但是默认情况下 AVPlayer 不支持音频下载功能。不过,我们可以通过一些方法来实现音频下载。
一种方法是使用 AVAssetExportSession,将音频文件导出为本地文件。具体步骤如下:
1. 创建 AVURLAsset 对象,用于获取音频资源。
2. 创建 AVAssetExportSession 对象,用于导出音频资源。
3. 设置 AVAssetExportSession 的输出路径和输出格式。
4. 调用 exportAsynchronouslyWithCompletionHandler 方法开始导出音频资源。
5. 在 completion handler 中获取导出的本地音频文件路径,然后使用 AVPlayer 播放该文件。
示例代码如下:
```objc
// 获取音频资源
AVURLAsset *asset = [AVURLAsset assetWithURL:[NSURL URLWithString:@"http://example.com/audio.mp3"]];
// 创建导出会话
AVAssetExportSession *exportSession = [AVAssetExportSession exportSessionWithAsset:asset presetName:AVAssetExportPresetAppleM4A];
// 设置输出路径和格式
NSString *outputPath = [NSTemporaryDirectory() stringByAppendingPathComponent:@"audio.m4a"];
exportSession.outputURL = [NSURL fileURLWithPath:outputPath];
exportSession.outputFileType = AVFileTypeAppleM4A;
// 开始导出
[exportSession exportAsynchronouslyWithCompletionHandler:^{
switch (exportSession.status) {
case AVAssetExportSessionStatusCompleted: {
// 获取导出的本地文件路径
NSString *localPath = exportSession.outputURL.path;
// 使用 AVPlayer 播放本地文件
AVPlayerItem *item = [[AVPlayerItem alloc] initWithURL:[NSURL fileURLWithPath:localPath]];
AVPlayer *player = [AVPlayer playerWithPlayerItem:item];
[player play];
break;
}
case AVAssetExportSessionStatusFailed:
NSLog(@"导出失败:%@", exportSession.error);
break;
default:
break;
}
}];
```
另一种方法是使用 AVAssetResourceLoaderDelegate,通过响应 NSURLSessionDataTask 的回调来实现音频下载。具体步骤如下:
1. 创建 AVURLAsset 对象,设置其 resourceLoader 的代理为自定义的 AVAssetResourceLoaderDelegate 对象。
2. 在代理对象中实现 resourceLoader:shouldWaitForLoadingOfRequestedResource: 方法,该方法会在播放器请求某个资源时被调用。
3. 在该方法中,创建一个 NSURLSessionDataTask 对象,使用该对象下载资源,并将下载完成的数据通过 AVAssetResourceLoadingRequest 的 respondWithData: 方法返回给播放器。
4. 在代理对象中实现 resourceLoader:didCancelLoadingRequest: 方法,该方法会在播放器不再需要请求某个资源时被调用,可以在该方法中取消 NSURLSessionDataTask 对象。
示例代码如下:
```objc
@interface CustomAVAssetResourceLoaderDelegate : NSObject<AVAssetResourceLoaderDelegate>
@property (nonatomic, strong) NSURLSession *session;
@end
@implementation CustomAVAssetResourceLoaderDelegate
- (BOOL)resourceLoader:(AVAssetResourceLoader *)resourceLoader shouldWaitForLoadingOfRequestedResource:(AVAssetResourceLoadingRequest *)loadingRequest {
// 创建 NSURLSessionDataTask 对象,下载资源
NSURLSessionDataTask *task = [self.session dataTaskWithURL:loadingRequest.request.URL completionHandler:^(NSData * _Nullable data, NSURLResponse * _Nullable response, NSError * _Nullable error) {
if (error) {
[loadingRequest finishLoadingWithError:error];
} else {
// 将下载完成的数据返回给播放器
[loadingRequest.dataRequest respondWithData:data];
[loadingRequest finishLoading];
}
}];
[task resume];
return YES;
}
- (void)resourceLoader:(AVAssetResourceLoader *)resourceLoader didCancelLoadingRequest:(AVAssetResourceLoadingRequest *)loadingRequest {
// 取消 NSURLSessionDataTask 对象
[self.session invalidateAndCancel];
}
@end
// 获取音频资源
AVURLAsset *asset = [AVURLAsset assetWithURL:[NSURL URLWithString:@"http://example.com/audio.mp3"]];
// 设置 resourceLoader 的代理为自定义的 AVAssetResourceLoaderDelegate 对象
CustomAVAssetResourceLoaderDelegate *delegate = [[CustomAVAssetResourceLoaderDelegate alloc] init];
delegate.session = [NSURLSession sessionWithConfiguration:[NSURLSessionConfiguration defaultSessionConfiguration]];
[asset.resourceLoader setDelegate:delegate queue:dispatch_get_main_queue()];
// 使用 AVPlayer 播放音频
AVPlayerItem *item = [[AVPlayerItem alloc] initWithAsset:asset];
AVPlayer *player = [AVPlayer playerWithPlayerItem:item];
[player play];
```
阅读全文
相关推荐
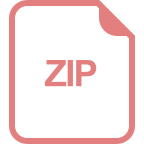
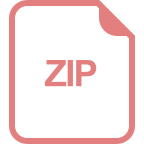















