java操作 docker 镜像获取manifest
时间: 2023-10-13 11:04:48 浏览: 177
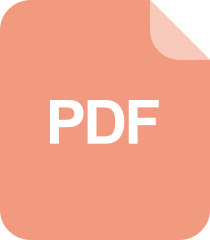
docker镜像命令
可以使用 Java 代码来获取 Docker 镜像的 manifest。以下是一个示例代码:
```java
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.Base64;
import org.apache.commons.codec.binary.Hex;
import org.apache.commons.io.IOUtils;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
public class DockerManifest {
public static void main(String[] args) throws NoSuchAlgorithmException, IOException {
String imageName = "nginx"; // 镜像名称
String imageTag = "latest"; // 镜像标签
String manifest = getManifest(imageName, imageTag);
System.out.println("Manifest: " + manifest);
}
public static String getManifest(String imageName, String imageTag)
throws NoSuchAlgorithmException, IOException {
String imageReference = imageName + ":" + imageTag;
String encodedReference = Base64.getUrlEncoder().encodeToString(imageReference.getBytes());
String endpoint = "https://registry-1.docker.io/v2";
String path = "/" + imageName + "/manifests/" + imageTag;
String url = endpoint + path;
MessageDigest md = MessageDigest.getInstance("SHA-256");
md.update(("GET\n\n\napplication/vnd.docker.distribution.manifest.v2+json\n" + "Accept: application/vnd.docker.distribution.manifest.v2+json\n" +
"Docker-Content-Digest:" + encodedReference + "\n" +
path).getBytes(StandardCharsets.UTF_8));
String digest = Hex.encodeHexString(md.digest());
// 发送请求
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(url)
.header("Accept", "application/vnd.docker.distribution.manifest.v2+json")
.header("Authorization", "Bearer " + getAuthToken())
.header("Docker-Content-Digest", encodedReference)
.header("Content-Type", "application/vnd.docker.distribution.manifest.v2+json")
.header("Digest", "sha256:" + digest)
.build();
Response response = client.newCall(request).execute();
String responseBody = response.body().string();
Gson gson = new GsonBuilder().setPrettyPrinting().create();
Object json = gson.fromJson(responseBody, Object.class);
return gson.toJson(json);
}
private static String getAuthToken() throws IOException {
String endpoint = "https://auth.docker.io/token";
String scope = "repository:library/*:pull";
String service = "registry.docker.io";
String url = endpoint + "?scope=" + scope + "&service=" + service;
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(url)
.build();
Response response = client.newCall(request).execute();
String responseBody = response.body().string();
Gson gson = new Gson();
AuthTokenResponse authTokenResponse = gson.fromJson(responseBody, AuthTokenResponse.class);
return authTokenResponse.getToken();
}
static class AuthTokenResponse {
private String token;
public String getToken() {
return token;
}
public void setToken(String token) {
this.token = token;
}
}
}
```
该代码使用了 okhttp 和 Gson 库来发送 HTTP 请求并解析 JSON 响应。您需要将 `imageName` 和 `imageTag` 替换为您自己的值。执行该代码后,将输出 Docker 镜像的 manifest。
阅读全文
相关推荐
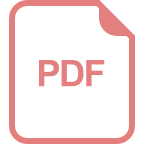

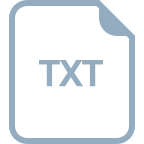
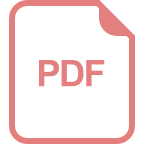
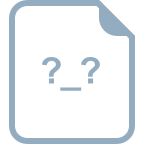
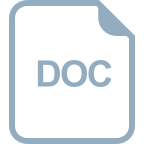
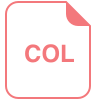
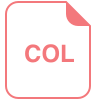
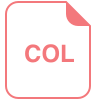
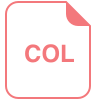
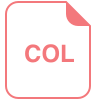






