opencv 两幅图像相减
时间: 2023-07-24 11:31:21 浏览: 349
在OpenCV中,可以使用subtract函数对两幅图像进行相减操作。具体步骤如下:
1. 加载需要相减的两幅图像,保证它们的大小和类型相同。
```python
import cv2
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
```
2. 对两幅图像进行相减操作,得到差值图像。
```python
diff = cv2.subtract(img1, img2)
```
注意:在相减操作中,如果差值为负数,OpenCV会将其截断为0。
3. 显示差值图像。
```python
cv2.imshow('difference', diff)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这样就可以对两幅图像进行相减操作,并显示出差值图像。
相关问题
python两幅遥感图像相减
在Python中,可以使用OpenCV和numpy库来实现两幅遥感图像的相减操作。下面是一个示例代码:
```python
import cv2
import numpy as np
# 读取两幅图像
image1 = cv2.imread('image1.jpg')
image2 = cv2.imread('image2.jpg')
# 将图像转换为灰度图像
gray1 = cv2.cvtColor(image1, cv2.COLOR_BGR2GRAY)
gray2 = cv2.cvtColor(image2, cv2.COLOR_BGR2GRAY)
# 将灰度图像转换为浮点型
gray1 = gray1.astype(float)
gray2 = gray2.astype(float)
# 图像相减
result = cv2.subtract(gray1, gray2)
# 将结果转换为8位无符号整型
result = np.abs(result).astype(np.uint8)
# 显示结果图像
cv2.imshow('Subtraction Result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
上述代码首先使用`cv2.imread`函数读取两幅图像,并使用`cv2.cvtColor`函数将其转换为灰度图像。然后,将灰度图像转换为浮点型,并使用`cv2.subtract`函数进行相减操作。最后,将结果转换为8位无符号整型,并使用`cv2.imshow`函数显示结果图像。
请注意,上述代码中的`image1.jpg`和`image2.jpg`是示例图像的文件名,你需要根据实际情况修改为你自己的图像文件名。
不使用opencv将膨胀后的图像和腐蚀后的图像相减c语言
不使用OpenCV库也可以实现膨胀和腐蚀操作,并且可以通过自定义函数实现对两幅图像的相减操作。以下是实现的示例代码:
```c++
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 膨胀操作
void dilate(unsigned char *src, unsigned char *dst, int width, int height)
{
int i, j, k, l;
unsigned char max_val;
for (i = 1; i < height - 1; i++)
{
for (j = 1; j < width - 1; j++)
{
max_val = 0;
for (k = -1; k <= 1; k++)
{
for (l = -1; l <= 1; l++)
{
if (src[(i + k) * width + j + l] > max_val)
{
max_val = src[(i + k) * width + j + l];
}
}
}
dst[i * width + j] = max_val;
}
}
}
// 腐蚀操作
void erode(unsigned char *src, unsigned char *dst, int width, int height)
{
int i, j, k, l;
unsigned char min_val;
for (i = 1; i < height - 1; i++)
{
for (j = 1; j < width - 1; j++)
{
min_val = 255;
for (k = -1; k <= 1; k++)
{
for (l = -1; l <= 1; l++)
{
if (src[(i + k) * width + j + l] < min_val)
{
min_val = src[(i + k) * width + j + l];
}
}
}
dst[i * width + j] = min_val;
}
}
}
// 图像相减操作
void subtract(unsigned char *src1, unsigned char *src2, unsigned char *dst, int width, int height)
{
int i, j;
for (i = 0; i < height; i++)
{
for (j = 0; j < width; j++)
{
dst[i * width + j] = abs(src1[i * width + j] - src2[i * width + j]);
}
}
}
int main()
{
// 读取原始图像
FILE *fp = fopen("lena.raw", "rb");
if (fp == NULL)
{
printf("读取图像失败!\n");
return -1;
}
int width = 512;
int height = 512;
unsigned char *srcImage = (unsigned char *)malloc(width * height * sizeof(unsigned char));
fread(srcImage, sizeof(unsigned char), width * height, fp);
fclose(fp);
// 将原始图像转化为灰度图像
unsigned char *grayImage = (unsigned char *)malloc(width * height * sizeof(unsigned char));
int i, j;
for (i = 0; i < height; i++)
{
for (j = 0; j < width; j++)
{
grayImage[i * width + j] = srcImage[i * width * 3 + j * 3] * 0.299 + srcImage[i * width * 3 + j * 3 + 1] * 0.587 + srcImage[i * width * 3 + j * 3 + 2] * 0.114;
}
}
// 对灰度图像进行膨胀操作
unsigned char *dilateImage = (unsigned char *)malloc(width * height * sizeof(unsigned char));
dilate(grayImage, dilateImage, width, height);
// 对灰度图像进行腐蚀操作
unsigned char *erodeImage = (unsigned char *)malloc(width * height * sizeof(unsigned char));
erode(grayImage, erodeImage, width, height);
// 对膨胀后的图像和腐蚀后的图像进行相减操作
unsigned char *subtractImage = (unsigned char *)malloc(width * height * sizeof(unsigned char));
subtract(dilateImage, erodeImage, subtractImage, width, height);
// 将差值图像保存为raw格式文件
fp = fopen("subtract.raw", "wb");
fwrite(subtractImage, sizeof(unsigned char), width * height, fp);
fclose(fp);
// 释放内存
free(srcImage);
free(grayImage);
free(dilateImage);
free(erodeImage);
free(subtractImage);
return 0;
}
```
在上述代码中,我们首先读取原始图像并将其转化为灰度图像,然后使用自定义的`dilate()`和`erode()`函数对灰度图像进行膨胀和腐蚀操作,最后使用自定义的`subtract()`函数对膨胀后的图像和腐蚀后的图像进行相减操作,得到差值图像。最后,我们将差值图像保存为raw格式文件。
阅读全文
相关推荐
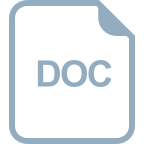
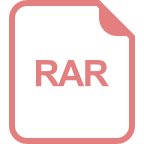
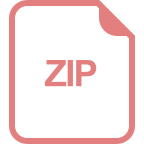



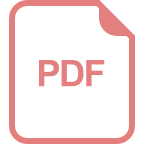
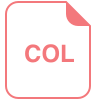
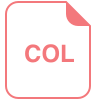
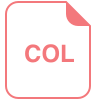
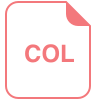
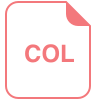
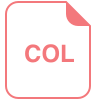
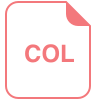