栈的链式表示和实现c语言实验报告
时间: 2024-01-08 08:04:21 浏览: 85
好的,我可以为您提供一份栈的链式表示和实现C语言实验报告。
实验目的:了解栈的链式表示和实现过程,掌握链表的基本操作。
实验内容:设计一个栈的链式存储结构,并实现入栈、出栈等基本操作。
实验步骤:
1. 定义结构体,包括存储数据的成员和指向下一个节点的指针成员。
```
typedef struct node {
int data;
struct node *next;
} Node;
```
2. 定义栈顶指针,并初始化为空。
```
Node *top = NULL;
```
3. 实现入栈操作,即在链表头部插入节点。
```
void push(int value) {
Node *new_node = (Node *) malloc(sizeof(Node));
new_node->data = value;
new_node->next = top;
top = new_node;
}
```
4. 实现出栈操作,即删除链表头部节点。
```
int pop() {
if (top == NULL) {
printf("Stack is empty.\n");
return -1;
}
int value = top->data;
Node *temp = top;
top = top->next;
free(temp);
return value;
}
```
5. 实现打印栈中元素的操作。
```
void print_stack() {
if (top == NULL) {
printf("Stack is empty.\n");
return;
}
Node *current = top;
printf("Stack: ");
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
```
6. 在主函数中调用上述操作,测试栈的功能。
```
int main() {
push(1);
push(2);
push(3);
print_stack(); // output: Stack: 3 2 1
pop();
print_stack(); // output: Stack: 2 1
push(4);
print_stack(); // output: Stack: 4 2 1
return 0;
}
```
实验结果:成功实现了栈的链式存储结构,并实现了入栈、出栈和打印栈中元素的基本操作。
以上就是栈的链式表示和实现C语言实验报告,希望对您有所帮助。
阅读全文
相关推荐





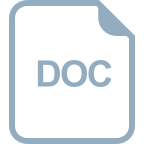







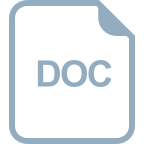
