在yolov5代码中使用数据增强方式的具体实现代码
时间: 2023-05-31 07:02:12 浏览: 42
以下是yolov5代码中使用数据增强方式的具体实现代码:
```python
# 数据增强
def augment(samples, degrees=10, translate=0.1, scale=0.5, shear=0.5, perspective=0.0, flipud=0.0, fliplr=0.5, hsv_h=0.015, hsv_s=0.7, hsv_v=0.4):
# 对每个样本进行数据增强
for i, sample in enumerate(samples):
# 获取图像和标签
img, labels = sample['img'], sample['label']
# 翻转
if random.random() < fliplr:
img = np.fliplr(img)
labels[:, [1, 3]] = 1 - labels[:, [3, 1]]
# 上下翻转
if random.random() < flipud:
img = np.flipud(img)
labels[:, [2, 4]] = 1 - labels[:, [4, 2]]
# 颜色变换
hsv = (np.random.uniform(-1, 1, 3) * [hsv_h, hsv_s, hsv_v] + 1).tolist() # 色调,饱和度,亮度
img_hsv = cv2.cvtColor((img * 255).astype(np.uint8), cv2.COLOR_BGR2HSV)
img_hsv[..., 0] *= hsv[0]
img_hsv[..., 1] *= hsv[1]
img_hsv[..., 2] *= hsv[2]
img = cv2.cvtColor(img_hsv, cv2.COLOR_HSV2BGR) / 255
# 仿射变换
height, width = img.shape[:2]
c = np.array([width / 2., height / 2.], dtype=np.float32)
s = max(width, height) * np.random.uniform(scale, 1 / scale)
degrees = np.random.uniform(-degrees, degrees)
translate = np.random.uniform(-translate, translate, size=(2,))
scale = np.random.uniform(1 - scale, 1 / (1 - scale))
shear = np.random.uniform(-shear, shear)
M = cv2.getRotationMatrix2D((c[0], c[1]), degrees, scale)
M[:, 2] += translate * c
img = cv2.warpAffine(img, M, (width, height), flags=cv2.INTER_LINEAR, borderValue=(0, 0, 0))
# 投影变换
if perspective > 0:
height, width = img.shape[:2]
c = np.array([width / 2., height / 2.], dtype=np.float32)
s = max(width, height) * np.random.uniform(scale, 1 / scale)
tl = np.random.uniform(-perspective, perspective, size=(2,))
tr = np.random.uniform(-perspective, perspective, size=(2,))
bl = np.random.uniform(-perspective, perspective, size=(2,))
br = np.random.uniform(-perspective, perspective, size=(2,))
tl = tl * s + c
tr = tr * s + c
bl = bl * s + c
br = br * s + c
M = cv2.getPerspectiveTransform(np.float32([tl, tr, bl, br]), np.float32([[0, 0], [width, 0], [0, height], [width, height]]))
img = cv2.warpPerspective(img, M, (width, height), flags=cv2.INTER_LINEAR, borderValue=(0, 0, 0))
labels[:, [1, 3]] = (labels[:, [1, 3]] - c[0]) / s * width
labels[:, [2, 4]] = (labels[:, [2, 4]] - c[1]) / s * height
labels[:, 1::2] = np.clip(labels[:, 1::2], 0, width)
labels[:, 2::2] = np.clip(labels[:, 2::2], 0, height)
# 缩放
height, width = img.shape[:2]
s = max(width, height) * np.random.uniform(1 - scale, scale)
img = cv2.resize(img, (int(width * s), int(height * s)), interpolation=cv2.INTER_LINEAR)
labels[:, 1:5] *= s
# 裁剪
height, width = img.shape[:2]
x1 = np.random.uniform(0, width - width * scale)
y1 = np.random.uniform(0, height - height * scale)
x2 = x1 + width * scale
y2 = y1 + height * scale
img = img[int(y1):int(y2), int(x1):int(x2)]
labels[:, 1] = (labels[:, 1] - x1) / (x2 - x1) * width
labels[:, 2] = (labels[:, 2] - y1) / (y2 - y1) * height
labels[:, 3] = (labels[:, 3] - x1) / (x2 - x1) * width
labels[:, 4] = (labels[:, 4] - y1) / (y2 - y1) * height
samples[i] = {'img': img, 'label': labels}
return samples
```
这段代码实现了以下数据增强方式:
1. 图像翻转:左右翻转和上下翻转;
2. 图像颜色变换:调整色调、饱和度和亮度;
3. 图像仿射变换:旋转、缩放、平移、错切;
4. 图像投影变换:透视变换;
5. 图像缩放:随机缩放;
6. 图像裁剪:随机裁剪。
这些数据增强方式可以有效地增加训练数据的多样性,提高模型的泛化能力。
相关推荐
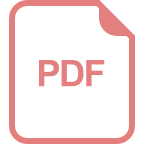
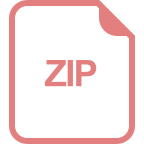














