python爬取csgo选手数据并可视化
时间: 2023-06-13 14:03:21 浏览: 381
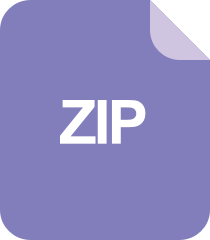
基于SpringBoot的CSGO赛事管理系统+lw.zip
要爬取 CSGO 选手数据,你需要了解如何从网页中获取数据。你可以使用 Python 中的 requests 和 BeautifulSoup 库来帮助你完成这个任务。以下是一个简单的爬取 CSGO 选手数据的示例代码:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 设置请求头
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"}
# 发送请求
url = "https://www.hltv.org/stats/players?startDate=2021-01-01&endDate=2021-12-31&matchType=BigEvents"
response = requests.get(url, headers=headers)
# 解析网页
soup = BeautifulSoup(response.content, "html.parser")
table = soup.find("table", class_="stats-table player-ratings-table")
# 将数据转换成 Pandas DataFrame
data = []
rows = table.find_all("tr")
for row in rows:
cols = row.find_all("td")
cols = [col.text.strip() for col in cols]
data.append(cols)
df = pd.DataFrame(data[1:], columns=data[0])
df = df.drop(columns=["#", "Unnamed: 0", "Maps"])
df = df.astype({"K-D Diff": int, "Rating": float})
# 保存数据
df.to_csv("csgo_player_data.csv", index=False)
```
这个代码将从 HLTV 网站的选手排名页面爬取 2021 年所有大型赛事的选手数据,并将数据存储到一个 CSV 文件中。
要可视化这些数据,你可以使用 Python 中的 Matplotlib 或 Seaborn 库。以下是一个简单的使用 Seaborn 绘制选手评分直方图的示例代码:
```python
import seaborn as sns
import pandas as pd
# 读取数据
df = pd.read_csv("csgo_player_data.csv")
# 绘制直方图
sns.histplot(data=df, x="Rating", bins=20)
```
这个代码将从之前生成的 CSV 文件中读取选手数据,并绘制评分直方图。你可以根据需要使用其他 Seaborn 或 Matplotlib 函数来绘制其他类型的图表,如散点图、线图等,以更好地可视化数据。
阅读全文
相关推荐
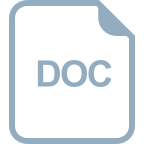
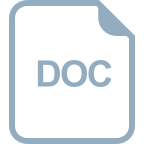


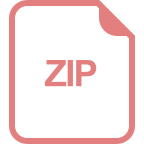
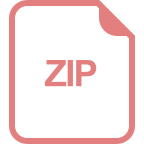
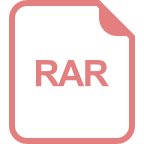
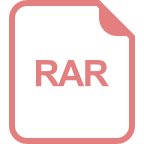
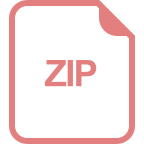
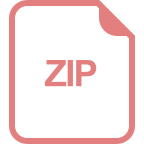







