编程进程或作业先来先服务、高优先权、按时间片轮转调度算法c语言版本
时间: 2023-08-06 08:07:51 浏览: 103
以下是三种调度算法的C语言实现:
1. 先来先服务(FCFS)调度算法:
```c
#include <stdio.h>
int main() {
int n, bt[20], wt[20], tat[20], i;
float wtAvg = 0, tatAvg = 0;
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time for each process: ");
for(i = 0; i < n; i++) {
scanf("%d", &bt[i]);
}
// Calculate waiting time for each process
wt[0] = 0;
for(i = 1; i < n; i++) {
wt[i] = wt[i-1] + bt[i-1];
}
// Calculate turnaround time for each process
for(i = 0; i < n; i++) {
tat[i] = wt[i] + bt[i];
}
// Calculate average waiting time and turnaround time
for(i = 0; i < n; i++) {
wtAvg += wt[i];
tatAvg += tat[i];
}
wtAvg /= n;
tatAvg /= n;
// Print the results
printf("Process\tBurst Time\tWaiting Time\tTurnaround Time\n");
for(i = 0; i < n; i++) {
printf("%d\t\t%d\t\t%d\t\t%d\n", i+1, bt[i], wt[i], tat[i]);
}
printf("Average waiting time: %.2f\n", wtAvg);
printf("Average turnaround time: %.2f\n", tatAvg);
return 0;
}
```
2. 高优先权调度算法:
```c
#include <stdio.h>
int main() {
int n, bt[20], wt[20], tat[20], p[20], i, j, temp;
float wtAvg = 0, tatAvg = 0;
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time and priority for each process:\n");
for(i = 0; i < n; i++) {
scanf("%d %d", &bt[i], &p[i]);
}
// Sort the processes based on priority
for(i = 0; i < n-1; i++) {
for(j = i+1; j < n; j++) {
if(p[i] > p[j]) {
temp = p[i];
p[i] = p[j];
p[j] = temp;
temp = bt[i];
bt[i] = bt[j];
bt[j] = temp;
}
}
}
// Calculate waiting time for each process
wt[0] = 0;
for(i = 1; i < n; i++) {
wt[i] = wt[i-1] + bt[i-1];
}
// Calculate turnaround time for each process
for(i = 0; i < n; i++) {
tat[i] = wt[i] + bt[i];
}
// Calculate average waiting time and turnaround time
for(i = 0; i < n; i++) {
wtAvg += wt[i];
tatAvg += tat[i];
}
wtAvg /= n;
tatAvg /= n;
// Print the results
printf("Process\tBurst Time\tPriority\tWaiting Time\tTurnaround Time\n");
for(i = 0; i < n; i++) {
printf("%d\t\t%d\t\t%d\t\t%d\t\t%d\n", i+1, bt[i], p[i], wt[i], tat[i]);
}
printf("Average waiting time: %.2f\n", wtAvg);
printf("Average turnaround time: %.2f\n", tatAvg);
return 0;
}
```
3. 时间片轮转调度算法:
```c
#include <stdio.h>
int main() {
int n, bt[20], wt[20], tat[20], rt[20], t, q, i, flag, done = 0;
float wtAvg = 0, tatAvg = 0;
printf("Enter the number of processes: ");
scanf("%d", &n);
printf("Enter the burst time for each process: ");
for(i = 0; i < n; i++) {
scanf("%d", &bt[i]);
rt[i] = bt[i];
}
printf("Enter the time quantum: ");
scanf("%d", &q);
// Run the processes until all are done
for(t = 0; done != n; t++) {
flag = 0;
for(i = 0; i < n; i++) {
if(rt[i] > 0) {
flag = 1;
if(rt[i] > q) {
t += q;
rt[i] -= q;
}
else {
t += rt[i];
wt[i] = t - bt[i];
rt[i] = 0;
done++;
}
}
}
if(flag == 0) {
break;
}
}
// Calculate turnaround time for each process
for(i = 0; i < n; i++) {
tat[i] = bt[i] + wt[i];
}
// Calculate average waiting time and turnaround time
for(i = 0; i < n; i++) {
wtAvg += wt[i];
tatAvg += tat[i];
}
wtAvg /= n;
tatAvg /= n;
// Print the results
printf("Process\tBurst Time\tWaiting Time\tTurnaround Time\n");
for(i = 0; i < n; i++) {
printf("%d\t\t%d\t\t%d\t\t%d\n", i+1, bt[i], wt[i], tat[i]);
}
printf("Average waiting time: %.2f\n", wtAvg);
printf("Average turnaround time: %.2f\n", tatAvg);
return 0;
}
```
阅读全文
相关推荐
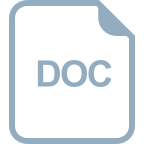
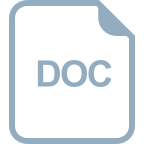
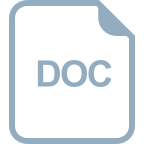




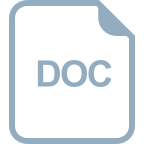
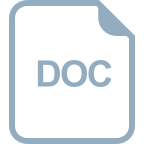
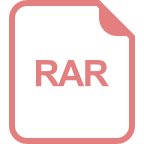
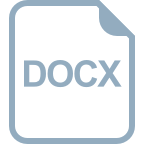
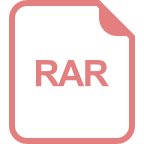
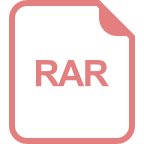
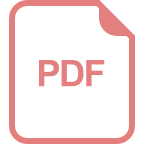
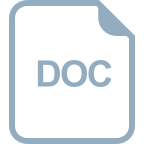



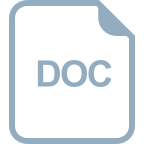