我在用putNextEntry放文件的时候,把文件名改了可以正常将文件写入压缩包么
时间: 2024-03-21 22:37:50 浏览: 102
可以的,您可以使用ZipEntry的构造函数来指定要在压缩文件中使用的文件名。例如,假设您要将文件“example.txt”压缩到压缩包中,并将其重命名为“newname.txt”,则可以按照以下方式创建ZipEntry对象:
```java
File file = new File("example.txt");
ZipEntry zipEntry = new ZipEntry("newname.txt");
zipOutputStream.putNextEntry(zipEntry);
FileInputStream fileInputStream = new FileInputStream(file);
byte[] buffer = new byte[1024];
int length;
while ((length = fileInputStream.read(buffer)) > 0) {
zipOutputStream.write(buffer, 0, length);
}
fileInputStream.close();
zipOutputStream.closeEntry();
```
在上面的示例中,您可以看到我们将ZipEntry的构造函数中的文件名设置为“newname.txt”。这将导致文件“example.txt”被压缩到压缩包中,并以“newname.txt”命名。请注意,在创建ZipEntry对象时,您可以指定任何您想要在压缩包中使用的文件名。
相关问题
帮我优化一下 这段代码 try (ZipOutputStream zipOut = new ZipOutputStream(response.getOutputStream())) { for (Map<String, String> iUrl : dbUrls) { for (Map.Entry<String, String> map : iUrl.entrySet()) { //检查是否有同名文件 String fileName = checkFileName(urlList, map.getKey()); URL url = new URL(map.getValue());// 读取url信息 zipOut.putNextEntry(new ZipEntry(fileName));// 创建url文件 //将文件名放入list,检查是否同名 urlList.add(map.getKey()); try { InputStream inputStream = url.openStream(); InputStream in = new BufferedInputStream(inputStream);// 读取url文件信息 zipOut.write(readInputStream(in));//把url文件写入zip中 in.close(); // 关闭连接 } catch (IOException e) { e.printStackTrace(); break; } } } zipOut.close(); zipOut.flush(); // zipOut.closeEntry();// 关闭入口 } catch (IOException e) { e.printStackTrace(); throw new Exception("导出压缩包失败"); }
这段代码中可以进行以下优化:
1. 对于文件读取和写入操作,可以使用NIO库中的Channels和Buffers来进行优化,可以提高读写速度和效率。
2. 对于InputStream的关闭操作,可以使用try-with-resources语句来简化代码和避免资源泄漏。
3. 在进行文件操作前,可以先对文件进行缓存,减少重复读写。
4. 对于异常处理,最好不要使用printStackTrace()方法,而是应该记录错误日志或者抛出自定义异常。
优化后的代码如下:
```java
try (ZipOutputStream zipOut = new ZipOutputStream(response.getOutputStream());
ReadableByteChannel inChannel = Channels.newChannel(inputStream);
) {
ByteBuffer buffer = ByteBuffer.allocate(1024);
for (Map<String, String> iUrl : dbUrls) {
for (Map.Entry<String, String> map : iUrl.entrySet()) {
//检查是否有同名文件
String fileName = checkFileName(urlList, map.getKey());
URL url = new URL(map.getValue());
zipOut.putNextEntry(new ZipEntry(fileName));
//将文件名放入list,检查是否同名
urlList.add(map.getKey());
try (InputStream inputStream = url.openStream();
ReadableByteChannel urlChannel = Channels.newChannel(inputStream);
) {
while (urlChannel.read(buffer) != -1) {
buffer.flip();
zipOut.write(buffer.array(), 0, buffer.limit());
buffer.clear();
}
} catch (IOException e) {
throw new Exception("导出压缩包失败");
}
zipOut.closeEntry();// 关闭入口
}
}
zipOut.flush();
} catch (IOException e) {
throw new Exception("导出压缩包失败");
}
```
注意,以上代码仅供参考,优化方案需要根据实际情况进行调整。
Java怎么修改压缩包里的文件 不要创建新文件
我们可以使用Java的ZipFile和ZipOutputStream类来完成这个任务。具体步骤如下:
1. 使用ZipFile类读取压缩包文件,获取需要修改的文件的输入流以及其在压缩包中的位置。
2. 使用ZipOutputStream类创建一个新的压缩包文件,并将需要修改的文件以及其他文件的输入流写入到该文件中。
3. 关闭输入流和输出流,删除原来的压缩包文件,将新的压缩包文件重命名为原来的文件名。
以下是示例代码:
```java
import java.io.*;
import java.util.Enumeration;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import java.util.zip.ZipOutputStream;
public class ModifyFileInZip {
public static void main(String[] args) throws IOException {
// 压缩包文件路径
String zipFilePath = "test.zip";
// 需要修改的文件名
String fileNameToModify = "file.txt";
// 修改后的文件内容
String newFileContent = "This is the modified content.";
// 读取压缩包文件
ZipFile zipFile = new ZipFile(zipFilePath);
// 创建一个新的压缩包文件
ZipOutputStream zipOutputStream = new ZipOutputStream(new FileOutputStream("new.zip"));
// 遍历原来的压缩包文件
Enumeration<? extends ZipEntry> entries = zipFile.entries();
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
// 如果是需要修改的文件,将其内容替换为新的内容
if (entry.getName().equals(fileNameToModify)) {
zipOutputStream.putNextEntry(new ZipEntry(entry.getName()));
byte[] buffer = newFileContent.getBytes();
zipOutputStream.write(buffer, 0, buffer.length);
zipOutputStream.closeEntry();
}
// 如果不是需要修改的文件,直接将其写入到新的压缩包文件中
else {
zipOutputStream.putNextEntry(new ZipEntry(entry.getName()));
InputStream inputStream = zipFile.getInputStream(entry);
byte[] buffer = new byte[1024];
int len;
while ((len = inputStream.read(buffer)) > 0) {
zipOutputStream.write(buffer, 0, len);
}
inputStream.close();
zipOutputStream.closeEntry();
}
}
// 关闭输入流和输出流
zipFile.close();
zipOutputStream.close();
// 删除原来的压缩包文件
File zipFileToDelete = new File(zipFilePath);
zipFileToDelete.delete();
// 将新的压缩包文件重命名为原来的文件名
File newZipFile = new File("new.zip");
newZipFile.renameTo(zipFileToDelete);
}
}
```
需要注意的是,上述代码仅供参考,具体实现可能需要根据实际情况进行修改。同时,操作压缩包文件时要注意文件的编码格式,否则可能会出现乱码等问题。
阅读全文
相关推荐
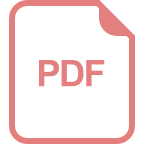
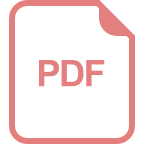













