java 不解压实现替换zip压缩包里面文件夹中的指定文件
时间: 2023-11-22 19:56:07 浏览: 398
Java提供了ZipInputStream和ZipOutputStream两个类来进行zip文件的读取和写入操作,可以不需要解压实现替换zip压缩包里面文件夹中的指定文件。以下是实现的步骤:
1. 创建ZipInputStream对象,读取zip文件中的内容。
2. 创建ZipOutputStream对象,向zip文件中写入内容。
3. 遍历ZipInputStream中的每一个ZipEntry,如果是要替换的文件,则跳过,否则将其写入ZipOutputStream中。
4. 将要替换的文件写入ZipOutputStream中。
5. 关闭ZipInputStream和ZipOutputStream对象。
以下是一个代码示例:
```java
import java.io.*;
import java.util.zip.*;
public class ReplaceFileInZip {
public static void main(String[] args) throws IOException {
String zipFilePath = "test.zip";
String fileNameToReplace = "test.txt";
String replacementFilePath = "replacement.txt";
// 创建ZipInputStream对象,读取zip文件中的内容
ZipInputStream zis = new ZipInputStream(new FileInputStream(zipFilePath));
// 创建ZipOutputStream对象,向zip文件中写入内容
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream("temp.zip"));
// 遍历ZipInputStream中的每一个ZipEntry,如果是要替换的文件,则跳过,否则将其写入ZipOutputStream中
ZipEntry entry;
while ((entry = zis.getNextEntry()) != null) {
if (entry.getName().equals(fileNameToReplace)) {
continue; // 不写入要替换的文件
}
zos.putNextEntry(new ZipEntry(entry.getName()));
byte[] buffer = new byte[1024];
int len;
while ((len = zis.read(buffer)) > 0) {
zos.write(buffer, 0, len);
}
zos.closeEntry();
}
// 将要替换的文件写入ZipOutputStream中
ZipEntry replacementEntry = new ZipEntry(fileNameToReplace);
zos.putNextEntry(replacementEntry);
FileInputStream fis = new FileInputStream(replacementFilePath);
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) > 0) {
zos.write(buffer, 0, len);
}
fis.close();
zos.closeEntry();
// 关闭ZipInputStream和ZipOutputStream对象
zis.close();
zos.close();
// 删除原来的zip文件并将新的temp.zip文件重命名为原来的文件名
new File(zipFilePath).delete();
new File("temp.zip").renameTo(new File(zipFilePath));
}
}
```
在上面的示例中,我们将test.zip文件中的test.txt文件替换为replacement.txt文件。注意,这个示例只是演示了如何进行zip文件中指定文件的替换,实际应用中还需要考虑异常处理、路径处理等问题。
阅读全文
相关推荐
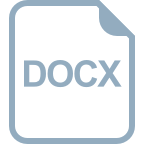

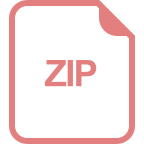
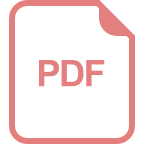
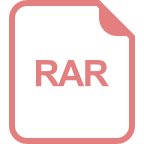
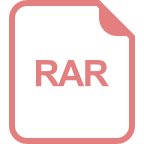
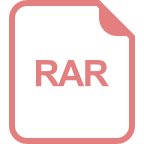
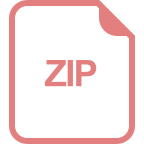
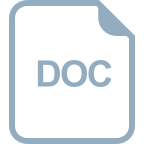
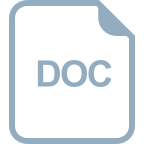
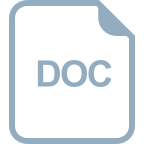
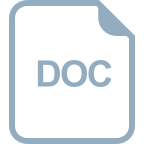
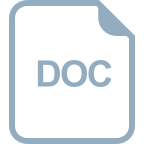
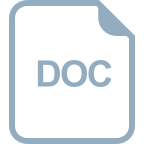
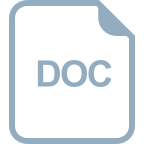
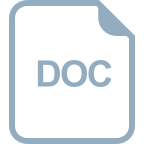
