The Sieve of Eratosthenes is a highly efficient way to generate a list of primes. It works by recognizing that all non-prime numbers are divisible by a prime number. We start with a list of all the numbers up through some value max. First, we cross off all numbers divisible by 2. Then, we look for the next prime (the next non-crossed off number) and cross off all numbers divisible by it. By crossing off all numbers divisible by 2, 3, 5, 7, 11, and so on, we wind up with a list of prime numbers from 2 through max. Write the code that implements the Sieve of Eratosthenes with the following prototype that returns an array of boolean values indicating if the corresponding integer is a prime number or not . Test the function with a main function . 用c++5.11寫出,且使用using namespace std;
时间: 2024-02-28 07:53:47 浏览: 247
以下是使用 C++ 实现 Sieve of Eratosthenes 的代码,返回一个布尔类型的数组,表示每个数是否为质数:
```c++
#include <iostream>
#include <vector>
using namespace std;
vector<bool> sieve_of_eratosthenes(int max) {
vector<bool> is_prime(max + 1, true);
is_prime[0] = is_prime[1] = false;
for (int i = 2; i * i <= max; i++) {
if (is_prime[i]) {
for (int j = i * i; j <= max; j += i) {
is_prime[j] = false;
}
}
}
return is_prime;
}
int main() {
int max = 100;
vector<bool> primes = sieve_of_eratosthenes(max);
for (int i = 2; i <= max; i++) {
if (primes[i]) {
cout << i << " ";
}
}
return 0;
}
```
在主函数中,我们调用 `sieve_of_eratosthenes` 函数,将 `max` 传递给它,并将返回的布尔类型向量存储在 `primes` 变量中。然后,我们遍历这个向量并打印所有值为 `true` 的索引,这些索引表示质数。
阅读全文
相关推荐
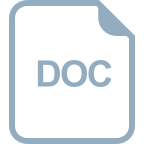
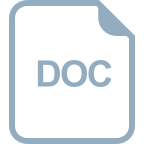
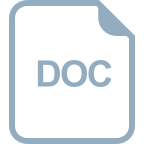
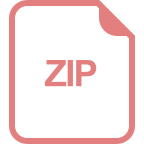
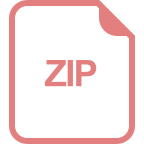
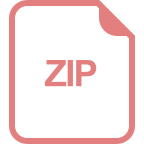
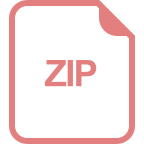
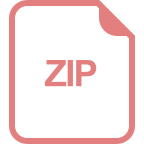
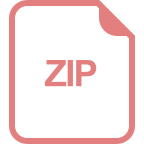
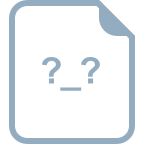
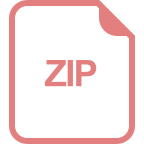
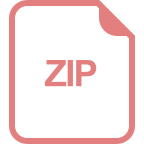
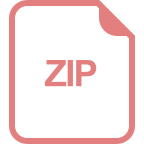
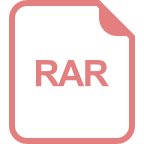
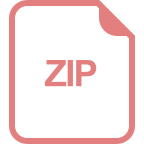
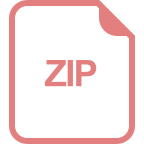
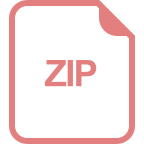

