写一个GUI编程界面的代码,创建窗体,设置为Borderlayout布局,放置5个按钮, 单独构造监听器类,实现点击按钮则在控制台中出现相应的提示。 提交相关代码
时间: 2024-06-12 07:08:17 浏览: 8
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class GUIProgram extends JFrame{
private JButton button1;
private JButton button2;
private JButton button3;
private JButton button4;
private JButton button5;
private JPanel panel;
public GUIProgram() {
super("Button Demo");
setSize(300, 200);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
setLayout(new BorderLayout());
button1 = new JButton("Button 1");
button2 = new JButton("Button 2");
button3 = new JButton("Button 3");
button4 = new JButton("Button 4");
button5 = new JButton("Button 5");
panel = new JPanel();
panel.add(button1);
panel.add(button2);
panel.add(button3);
panel.add(button4);
panel.add(button5);
add(panel, BorderLayout.CENTER);
button1.addActionListener(new ButtonListener());
button2.addActionListener(new ButtonListener());
button3.addActionListener(new ButtonListener());
button4.addActionListener(new ButtonListener());
button5.addActionListener(new ButtonListener());
}
private class ButtonListener implements ActionListener {
public void actionPerformed(ActionEvent e) {
if (e.getSource() == button1) {
System.out.println("You clicked button 1");
} else if (e.getSource() == button2) {
System.out.println("You clicked button 2");
} else if (e.getSource() == button3) {
System.out.println("You clicked button 3");
} else if (e.getSource() == button4) {
System.out.println("You clicked button 4");
} else if (e.getSource() == button5) {
System.out.println("You clicked button 5");
}
}
}
public static void main(String[] args) {
GUIProgram gui = new GUIProgram();
gui.setVisible(true);
}
}
相关推荐
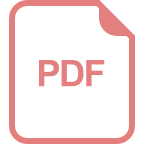
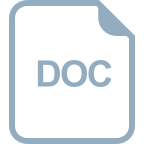
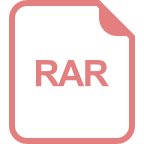









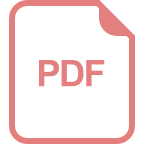
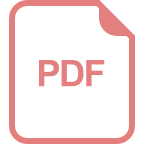
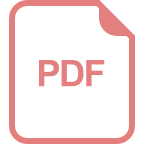
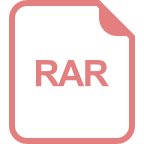
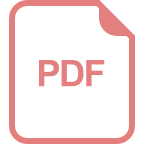
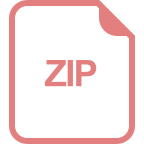