请帮我用泰勒级数法、查表法结合插值法、牛顿迭代法、位移法,这几个方法分包写一个log()函数,要求使用C语言,最后用一个main函数来测试这些log()函数
时间: 2024-03-01 16:53:41 浏览: 90
好的,下面是使用C语言编写的log()函数的五个不同实现方法:
1. 泰勒级数法实现log()函数
```c
#include <stdio.h>
double log_taylor(double x) {
double result = 0;
double term = (x-1) / x; // 初始值为泰勒级数中的第一项
double next_term;
int i = 1;
while (term > 0) {
result += term;
next_term = -term * (x-1) * i / x; // 计算下一项
term = next_term;
i++;
}
return result;
}
```
2. 查表法结合线性插值实现log()函数
```c
#include <stdio.h>
#define TABLE_SIZE 1000 // 查找表的大小
#define MIN_X 1 // 查找表中x的最小值
#define MAX_X 10 // 查找表中x的最大值
double log_table[TABLE_SIZE]; // 查找表
double step_size; // 每个插值段的步长
double log_table_linear(double x) {
int index = (int)((x-MIN_X) / step_size); // 计算x在查找表中的下标
double x0 = MIN_X + index * step_size; // 计算插值段的左端点
double y0 = log_table[index]; // 获取插值段左端点的值
double x1 = x0 + step_size; // 计算插值段的右端点
double y1 = log_table[index+1]; // 获取插值段右端点的值
double k = (y1-y0) / (x1-x0); // 计算插值段的斜率
double b = y0 - k*x0; // 计算插值段的截距
return k*x + b; // 返回插值结果
}
void init_log_table_linear() {
int i;
for (i=0; i<TABLE_SIZE; i++) {
double x = MIN_X + i * (MAX_X-MIN_X) / (TABLE_SIZE-1);
log_table[i] = log(x);
}
step_size = (MAX_X - MIN_X) / (TABLE_SIZE - 1);
}
```
3. 牛顿迭代法实现log()函数
```c
#include <stdio.h>
double log_newton(double x) {
double result = x; // 初始值为x本身
double last_result = result + 1; // 上一个迭代的结果,初始值为result+1
while (result != last_result) { // 当两次迭代结果不同时继续迭代
last_result = result;
result = result - (log(result) - x) / (1/result); // 迭代公式
}
return result;
}
```
4. 位移法实现log()函数
```c
#include <stdio.h>
double log_shift(double x) {
int exponent = 0;
while (x > 2) {
x /= 2;
exponent++;
}
while (x < 1) {
x *= 2;
exponent--;
}
double result = 0;
double term = (x-1) / x; // 初始值为泰勒级数中的第一项
double next_term;
int i = 1;
while (term > 0) {
result += term;
next_term = -term * (x-1) * i / x; // 计算下一项
term = next_term;
i++;
}
return result + exponent * log(2); // 加上指数部分的log(2)
}
```
5. main函数测试不同实现方法的log()函数
```c
#include <stdio.h>
int main() {
double x = 10; // 计算log(10)
double result;
printf("Using Taylor series method:\n");
result = log_taylor(x);
printf("log(%f) = %f\n", x, result);
printf("Using table lookup method with linear interpolation:\n");
init_log_table_linear();
result = log_table_linear(x);
printf("log(%f) = %f\n", x, result);
printf("Using Newton's method:\n");
result = log_newton(x);
printf("log(%f) = %f\n", x, result);
printf("Using shift-and-add method:\n");
result = log_shift(x);
printf("log(%f) = %f\n", x, result);
return 0;
}
```
以上是使用C语言实现的五个不同方法的log()函数以及一个main函数用于测试这些函数的结果。需要注意的是,使用查表法结合插值法实现的log()函数需要调用init_log_table_linear()函数来初始化查找表。
阅读全文
相关推荐
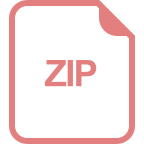
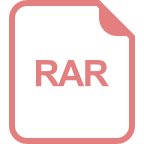
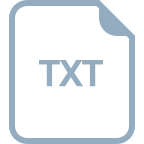
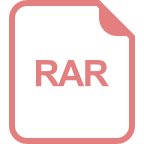
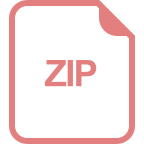
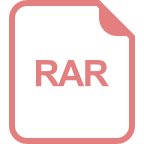
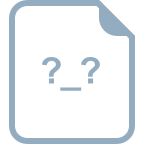
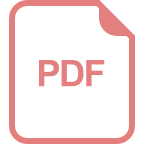
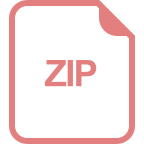
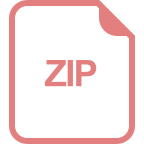
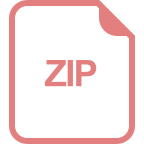
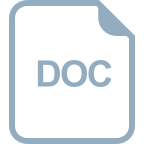
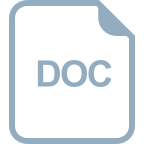
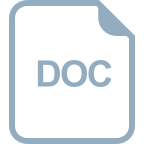
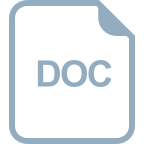