C++代码要求:1、建立一个二叉树。 2、对建立的二叉树进行遍历。 3、求二叉树叶子数和深度等应用。
时间: 2023-06-19 13:04:49 浏览: 51
以下是C++实现建立二叉树、遍历二叉树、求二叉树叶子数和深度的代码:
```c++
#include <iostream>
#include <queue>
using namespace std;
// 定义二叉树节点结构体
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
};
// 建立二叉树函数
void createTree(TreeNode*& root) {
int val;
cin >> val;
if (val == -1) { // 如果输入-1,则表示该节点为空
root = nullptr;
} else {
root = new TreeNode(val);
createTree(root->left);
createTree(root->right);
}
}
// 先序遍历
void preOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
cout << root->val << " ";
preOrder(root->left);
preOrder(root->right);
}
// 中序遍历
void inOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
inOrder(root->left);
cout << root->val << " ";
inOrder(root->right);
}
// 后序遍历
void postOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
postOrder(root->left);
postOrder(root->right);
cout << root->val << " ";
}
// 层序遍历
void levelOrder(TreeNode* root) {
if (root == nullptr) {
return;
}
queue<TreeNode*> q;
q.push(root);
while (!q.empty()) {
TreeNode* cur = q.front();
q.pop();
cout << cur->val << " ";
if (cur->left != nullptr) {
q.push(cur->left);
}
if (cur->right != nullptr) {
q.push(cur->right);
}
}
}
// 求二叉树叶子节点数
int countLeaves(TreeNode* root) {
if (root == nullptr) {
return 0;
}
if (root->left == nullptr && root->right == nullptr) {
return 1;
}
return countLeaves(root->left) + countLeaves(root->right);
}
// 求二叉树深度
int depth(TreeNode* root) {
if (root == nullptr) {
return 0;
}
int leftDepth = depth(root->left);
int rightDepth = depth(root->right);
return max(leftDepth, rightDepth) + 1;
}
int main() {
TreeNode* root = nullptr;
createTree(root);
cout << "先序遍历:";
preOrder(root);
cout << endl;
cout << "中序遍历:";
inOrder(root);
cout << endl;
cout << "后序遍历:";
postOrder(root);
cout << endl;
cout << "层序遍历:";
levelOrder(root);
cout << endl;
cout << "叶子节点数:" << countLeaves(root) << endl;
cout << "二叉树深度:" << depth(root) << endl;
return 0;
}
```
输入格式为一串二叉树前序遍历序列,其中空节点用-1表示。例如输入样例:
```
1 2 4 -1 -1 5 -1 -1 3 -1 -1
```
输出结果为:
```
先序遍历:1 2 4 5 3
中序遍历:4 2 5 1 3
后序遍历:4 5 2 3 1
层序遍历:1 2 3 4 5
叶子节点数:2
二叉树深度:3
```
相关推荐
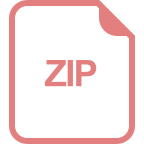
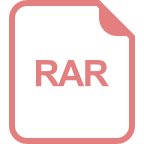














