如何在Vue2 中实现高德地图的搜索功能搜索地图
时间: 2024-02-23 15:33:22 浏览: 97
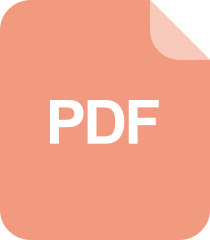
vue+高德地图实现地图搜索及点击定位操作
要在Vue2中实现高德地图的搜索功能,可以按照以下步骤操作:
1. 安装高德地图JavaScript API
在Vue2项目中,可以通过npm安装高德地图JavaScript API:
```bash
npm install @amap/amap-jsapi-loader --save
```
2. 创建高德地图组件
在Vue2项目中,可以创建一个高德地图组件,用于显示地图和搜索结果:
```vue
<template>
<div id="map-container"></div>
</template>
<script>
import { AMapLoader } from '@amap/amap-jsapi-loader';
export default {
name: 'MapContainer',
props: {
apiKey: String, // 高德地图API的key
searchKeywords: String, // 搜索关键字
},
data() {
return {
map: null, // 地图对象
markers: [], // 地图标记
};
},
mounted() {
this.initMap();
},
methods: {
async initMap() {
const loader = new AMapLoader({
key: this.apiKey,
version: '2.0',
});
const { AMap } = await loader.load();
this.map = new AMap.Map('map-container');
if (this.searchKeywords) {
this.search(this.searchKeywords);
}
},
async search(keywords) {
const { AMap } = await new AMapLoader({
key: this.apiKey,
version: '2.0',
}).load();
const placeSearch = new AMap.PlaceSearch({
map: this.map,
});
placeSearch.search(keywords, (status, result) => {
if (status === 'complete' && result.info === 'OK') {
this.markers = result.poiList.pois.map((poi) => {
const marker = new AMap.Marker({
position: poi.location,
title: poi.name,
});
marker.setMap(this.map);
return marker;
});
}
});
},
},
watch: {
searchKeywords(newVal) {
this.search(newVal);
},
},
};
</script>
<style>
#map-container {
width: 100%;
height: 100%;
}
</style>
```
3. 在父组件中使用高德地图组件
在父组件中,可以使用上述高德地图组件,并传入高德地图API的key和搜索关键字:
```vue
<template>
<div>
<input v-model="searchKeywords" placeholder="请输入关键字搜索地图" />
<MapContainer apiKey="your_amap_api_key" :searchKeywords="searchKeywords" />
</div>
</template>
<script>
import MapContainer from './MapContainer.vue';
export default {
name: 'App',
components: {
MapContainer,
},
data() {
return {
searchKeywords: '',
};
},
};
</script>
```
这样就可以在Vue2中实现高德地图的搜索功能。用户在输入框中输入关键字后,组件会自动搜索地图,并在地图上显示搜索结果。
阅读全文
相关推荐
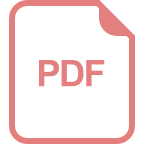















