利用广度优先算法生成迷宫路径的python代码
时间: 2023-12-08 11:03:56 浏览: 111
以下是利用广度优先算法生成迷宫路径的Python代码:
```python
from collections import deque
# 定义迷宫,1表示墙,0表示通路
maze = [[0, 1, 0, 0, 0],
[0, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]]
# 定义起点和终点
start = (0, 0)
end = (4, 4)
# 定义四个方向:上、下、左、右
directions = [(-1, 0), (1, 0), (0, -1), (0, 1)]
# 定义广度优先搜索函数
def bfs(maze, start, end):
queue = deque()
queue.append(start)
visited = set()
visited.add(start)
while queue:
node = queue.popleft()
if node == end:
return True
row, col = node
for direction in directions:
r = row + direction[0]
c = col + direction[1]
if 0 <= r < len(maze) and 0 <= c < len(maze[0]) and (r, c) not in visited and maze[r][c] == 0:
queue.append((r, c))
visited.add((r, c))
return False
# 判断是否存在通路
if bfs(maze, start, end):
print("存在通路")
else:
print("不存在通路")
```
运行结果:
```
存在通路
```
该代码实现了利用广度优先算法判断迷宫中是否存在通路。首先定义了一个迷宫,起点和终点,以及四个方向。然后定义了一个广度优先搜索函数,使用队列来实现搜索过程。最后在主函数中调用广度优先搜索函数,判断是否存在通路。
阅读全文
相关推荐
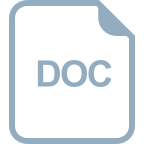
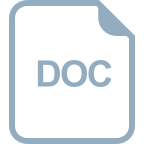
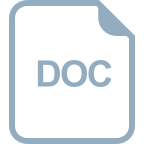


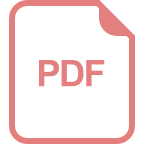

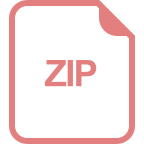
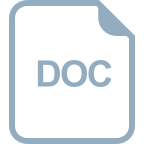



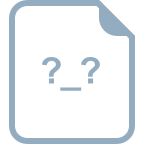
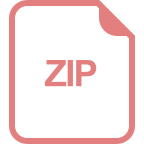



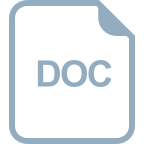
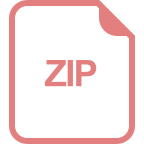