python用广度优先算法求解迷宫问题的代码
时间: 2024-01-05 08:02:52 浏览: 91
以下是Python实现的广度优先算法求解迷宫问题的代码,代码中maze是一个二维数组,0表示可通行,1表示障碍物。
```python
from queue import Queue
def bfs(maze, start, end):
q = Queue()
visited = set()
q.put(start)
visited.add(start)
while not q.empty():
cur_pos = q.get()
if cur_pos == end:
return True
for dir in [(-1, 0), (1, 0), (0, -1), (0, 1)]:
next_pos = (cur_pos[0] + dir[0], cur_pos[1] + dir[1])
if next_pos[0] < 0 or next_pos[0] >= len(maze) \
or next_pos[1] < 0 or next_pos[1] >= len(maze[0]):
continue
if next_pos in visited or maze[next_pos[0]][next_pos[1]] == 1:
continue
q.put(next_pos)
visited.add(next_pos)
return False
maze = [[0, 0, 1, 0],
[0, 0, 0, 0],
[0, 0, 1, 0],
[0, 1, 0, 0],
[0, 0, 0, 1]]
start = (0, 0)
end = (4, 3)
if bfs(maze, start, end):
print("Path found!")
else:
print("Path not found!")
```
当迷宫有解时,输出"Path found!",否则输出"Path not found!"。
阅读全文
相关推荐
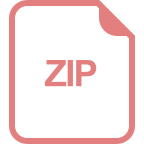
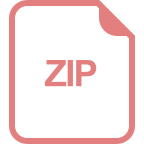
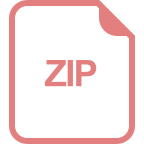















