a*算法求解迷宫寻路p代码
时间: 2024-12-23 10:10:52 浏览: 29
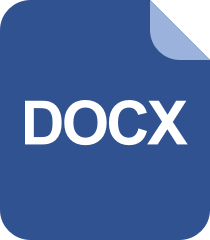
实验3 A实验求解迷宫码实验.docx
A*(A-star)算法是一种启发式搜索算法,用于寻找从起点到目标点的最短路径,常用于解决迷宫寻路问题。它结合了宽度优先搜索(BFS)的广度优先探索特性以及Dijkstra算法的贪心策略。以下是Python中使用A*算法求解迷宫寻路的一个基本代码示例:
```python
import heapq
# 定义节点类
class Node:
def __init__(self, x, y, cost=0, parent=None):
self.x = x
self.y = y
self.cost = cost
self.parent = parent
self.f_score = cost + heuristic(x, y, goal_x, goal_y) # f_score = g_score + h_score
def heuristic(a, b, c, d): # 估价函数,这里假设曼哈顿距离
return abs(a - c) + abs(b - d)
def a_star_search(maze, start, end):
open_set = []
heapq.heappush(open_set, (start.f_score, start))
came_from = {start: None}
while open_set:
current = heapq.heappop(open_set)[1]
if current == end:
path = []
while current is not None:
path.append((current.x, current.y))
current = came_from[current]
return path[::-1] # 返回路径从终点逆序到起点
for neighbor in neighbors(maze, current):
tentative_g_score = current.cost + 1 # 每次移动成本加一
if neighbor in came_from and tentative_g_score >= came_from[neighbor].cost:
continue # 如果已经访问过并有更短路径,则跳过
if neighbor not in open_set or tentative_g_score < open_set[0][0]:
open_set.remove(neighbor if neighbor in open_set else None)
neighbor.g_score = tentative_g_score
neighbor.f_score = tentative_g_score + heuristic(neighbor.x, neighbor.y, end.x, end.y)
neighbor.parent = current
heapq.heappush(open_set, (neighbor.f_score, neighbor))
return None # 如果找不到路径,返回None
def neighbors(maze, node):
possible_neighbors = [(node.x+1, node.y), (node.x-1, node.y),
(node.x, node.y+1), (node.x, node.y-1)]
valid_neighbors = [n for n in possible_neighbors if 0 <= n[0] < maze.shape[0] and 0 <= n[1] < maze.shape[1]]
return [Node(*n, maze[n[0]][n[1]]) for n in valid_neighbors if maze[n[0]][n[1]] != 1] # 避免走到墙壁
# 使用方法:
maze = ... # 迷宫矩阵,值1表示墙,0表示可以通行
start = Node(start_x, start_y) # 起点坐标
end = Node(end_x, end_y) # 目标坐标
path = a_star_search(maze, start, end)
```
在这个代码里,你需要提供一个迷宫矩阵`maze`,定义开始位置`start`和结束位置`end`,然后调用`a_star_search`函数计算路径。注意,这个版本仅适用于二维迷宫,并且采用曼哈顿距离作为启发函数。
阅读全文
相关推荐
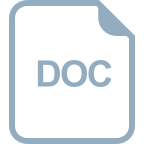
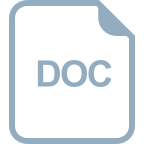

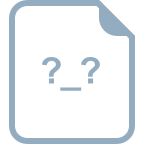
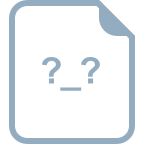











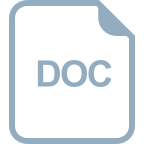