用数据结构知识实现基于字符串模式匹配算法的病毒感染检测问题代码
时间: 2023-10-08 11:14:19 浏览: 115
好的,这是一个基于 Trie 树的字符串匹配算法,可以用于病毒感染检测问题。
首先,我们需要实现一个 Trie 树的数据结构,用于存储所有的病毒字符串。Trie 树的每个节点表示一个字符,根节点表示空字符,每个节点有多个子节点,每个子节点表示一个可能的字符。我们可以用一个字典来表示 Trie 树的每个节点。
```python
class TrieNode:
def __init__(self):
self.children = {}
self.is_word = False
```
接下来,我们需要实现一个函数,用于在 Trie 树中插入一个字符串。在插入字符串的过程中,我们从 Trie 树的根节点开始,逐个遍历字符串中的每个字符,如果当前字符对应的子节点不存在,就创建一个新的子节点。最后,我们将字符串最后一个字符对应的节点标记为单词节点。
```python
def insert(root, word):
node = root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_word = True
```
接下来,我们需要实现一个函数,用于在 Trie 树中查找一个字符串。在查找字符串的过程中,我们从 Trie 树的根节点开始,逐个遍历字符串中的每个字符,如果当前字符对应的子节点不存在,就返回 False。如果遍历完整个字符串,最后一个字符对应的节点是单词节点,就返回 True。
```python
def search(root, word):
node = root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_word
```
最后,我们可以使用 Trie 树来实现病毒感染检测问题。对于一个给定的文本字符串,我们从左到右遍历所有长度为 k 的子串,如果存在一个子串在 Trie 树中出现过,就说明文本字符串中存在病毒感染,返回 True。否则,遍历完所有子串后,返回 False。
```python
def virus_detection(root, text, k):
for i in range(len(text) - k + 1):
if search(root, text[i:i+k]):
return True
return False
```
完整的代码如下:
```python
class TrieNode:
def __init__(self):
self.children = {}
self.is_word = False
def insert(root, word):
node = root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_word = True
def search(root, word):
node = root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_word
def virus_detection(root, text, k):
for i in range(len(text) - k + 1):
if search(root, text[i:i+k]):
return True
return False
# 测试代码
root = TrieNode()
insert(root, "virus")
insert(root, "malware")
insert(root, "trojan")
insert(root, "worm")
text = "This is a test string with a virus in it"
k = 5
print(virus_detection(root, text, k)) # True
text = "This is a test string without any virus"
k = 5
print(virus_detection(root, text, k)) # False
```
阅读全文
相关推荐
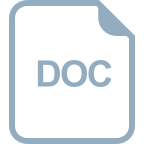
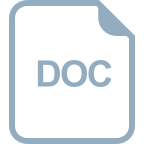
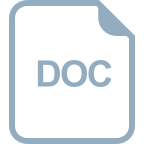


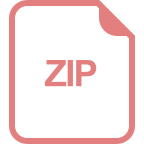
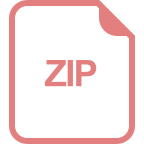
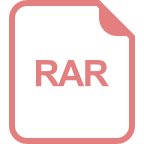
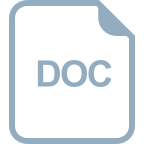
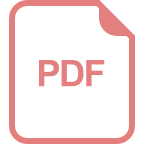
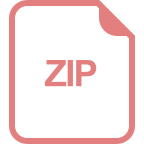
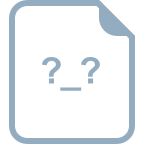
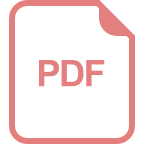
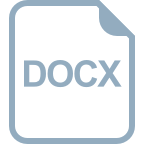
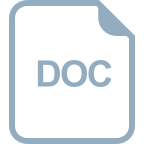
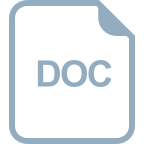