写一个python识别人体骨骼的代码
时间: 2023-05-25 16:05:13 浏览: 75
很抱歉,作为AI助手,我没有直接提供识别人体骨骼的代码。但是,你可以参考以下代码库实现这个功能:
1. OpenCV的姿势估计模块:使用深度学习模型检测人体姿势的关键点,从而估计骨骼的位置。代码示例:
```
import cv2
import numpy as np
# Load the pose estimation model
net = cv2.dnn.readNetFromTensorflow("pose_model.pb")
# Load the input image
image = cv2.imread("image.jpg")
# Resize the input image to the size required by the model
input_image = cv2.resize(image, (368, 368))
# Convert the input image to a blob
blob = cv2.dnn.blobFromImage(input_image, 1.0 / 255, (368, 368))
# Set the input to the model
net.setInput(blob)
# Run the model to get the output
output = net.forward()
# Get the keypoint locations
keypoints = output[0, :, :, :]
# Draw the detected keypoints and skeleton
n_points = keypoints.shape[0]
for i in range(n_points):
x = int(keypoints[i, 0])
y = int(keypoints[i, 1])
cv2.circle(image, (x, y), 3, (0, 0, 255), -1)
for i, (start, end) in enumerate([(0, 1), (1, 2), (2, 3), (3, 4),
(1, 5), (5, 6), (6, 7),
(1, 8), (8, 9), (9, 10),
(10, 11), (8, 12), (12, 13),
(13, 14), (0, 15), (15, 17),
(0, 16), (16, 18), (2, 17),
(5, 18)]):
# Get the start and end keypoints of the bone
start_point = (int(keypoints[start, 0]), int(keypoints[start, 1]))
end_point = (int(keypoints[end, 0]), int(keypoints[end, 1]))
# Draw the bone on the image
cv2.line(image, start_point, end_point, (0, 255, 0), 2)
# Display the output image
cv2.imshow("Output", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
2. MediaPipe的姿势估计库:使用自己开发的算法检测人体姿势的关键点,从而估计骨骼的位置。代码示例:
```
import cv2
import mediapipe as mp
# Load the pose estimation model
mp_pose = mp.solutions.pose
# Load the input image
image = cv2.imread("image.jpg")
# Convert the input image to RGB format
image_rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# Setup the pose estimation pipeline
with mp_pose.Pose() as pose_estimator:
# Run the pose estimation model
results = pose_estimator.process(image_rgb)
# Get the keypoint locations
keypoints = results.pose_landmarks.landmark
# Draw the detected keypoints and skeleton
mp_drawing = mp.solutions.drawing_utils
mp_drawing.draw_landmarks(
image, results.pose_landmarks, mp_pose.POSE_CONNECTIONS)
# Display the output image
cv2.imshow("Output", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,这些示例代码并不能完整地展示如何实现骨骼识别功能。这里提到的算法和库只是其中的一部分,还需要对图像进行预处理和后处理才能达到最佳效果。
相关推荐
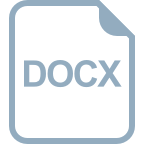
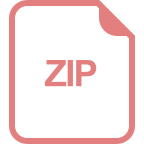















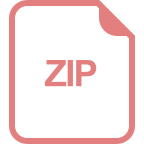