golang打印word
时间: 2023-07-26 09:17:21 浏览: 198
在Golang中打印Word文档,可以使用Microsoft Office提供的COM接口来实现。需要使用golang中的COM库github.com/go-ole/go-ole。
以下是一个简单的示例代码,可以在Windows平台下使用Golang语言和Microsoft Office COM接口打印Word文档:
```go
package main
import (
"fmt"
"github.com/go-ole/go-ole"
"github.com/go-ole/go-ole/oleutil"
)
func printWordDocument(filename string, printerName string) {
// 初始化COM库
ole.CoInitialize(0)
defer ole.CoUninitialize()
// 创建Word应用程序对象
unknown, err := oleutil.CreateObject("Word.Application")
if err != nil {
fmt.Println("Failed to create Word application instance.")
return
}
defer unknown.Release()
wordApp, err := unknown.QueryInterface(ole.IID_IDispatch)
if err != nil {
fmt.Println("Failed to create Word application instance.")
return
}
defer wordApp.Release()
// 设置打印机名称
_, err = oleutil.PutProperty(wordApp, "ActivePrinter", printerName)
if err != nil {
fmt.Println("Failed to set printer name.")
return
}
// 打开Word文档
documents := oleutil.MustGetProperty(wordApp, "Documents").ToIDispatch()
doc, err := oleutil.CallMethod(documents, "Open", filename)
if err != nil {
fmt.Println("Failed to open Word document.")
return
}
defer doc.ToIDispatch().Release()
// 打印文档
_, err = oleutil.CallMethod(doc, "PrintOut")
if err != nil {
fmt.Println("Failed to print Word document.")
return
}
fmt.Println("Printed Word document successfully.")
}
```
在上面的代码中,我们定义了一个printWordDocument函数,可以传入要打印的Word文档文件名和打印机名称,然后使用COM接口调用Microsoft Word应用程序来打印文档。我们首先使用CreateObject方法创建Word应用程序对象,然后打开指定的文档并使用PrintOut方法打印文档。请注意,需要安装Microsoft Office并将代码编译为Windows可执行文件。
阅读全文
相关推荐
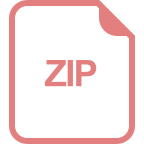
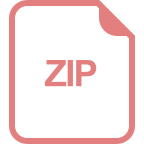

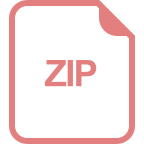
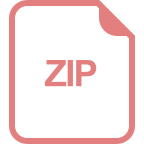
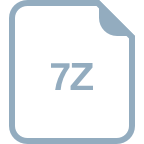
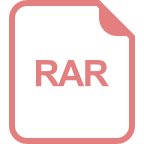
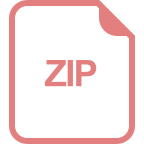
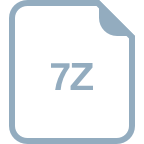
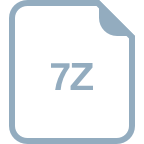
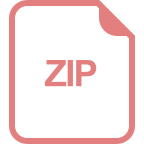
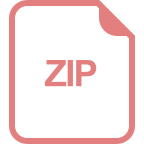
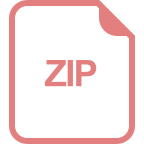
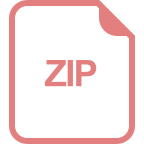
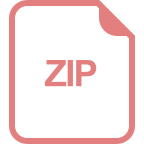
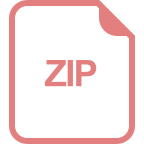