完善代码1.冒泡排序 2.快速排序 3.堆排序#include <iostream> #include<cstdlib> #include<Windows.h> #include<ctime> using namespace std; void randomAssign(int a[],int n){ srand(time(0)); for(int i = 0; i < n;i++) a[i] = rand()%n+1; } void print(const char* str,int a[],int n){ cout<<str<<"="; for(int i = 0; i < n; i++) cout<<a[i] <<" "; cout<<endl; } void bubbleSort(int a[],int n){ for(int i = n; i >= 1; i--) for(int j = 1; j < i; j++) if(a[j+1] > a[j]) swap(a[j+1],a[j]); } int onePartition(int a[],int left,int right){ int pivot = a[left]; int i = left; int j = right; while(i < j){ while(XXXXXXXXXXXXX;) j++; if( i < j) a[i] = a[j]; XXXXXXXXXXXXX; XXXXXXXXXXXXX; if( i < j) a[j] = a[i]; } XXXXXXXXXXXXX; return i; } void quickSort(int a[],int left,int right){ if(left >= right) return ; int pos = XXXXXXXXXXXXX;; XXXXXXXXXXXXX; quickSort(a,pos+1,right); } void quickSort(int a[],int n){ quickSort(a,0,n-1); } void sift(int a[],int pos,int n){ //n表示长度 int parent = pos; int son = parent * 2 + 1; int tmp = a[parent]; while (son <= n - 1){ // 有效下标 0...n-1 if (son + 1 <= n - 1 && a[son] < a[son + 1]) son++; if(XXXXXXXXXXXXX) return; else { XXXXXXXXXXXXX; parent = son; son = parent * 2 + 1; } } } void heapSort(int a[],int n) { int i = 0; for (i = n / 2 - 1; i >= 0; i--) //从最后一个非叶结点开始 sift(a,i,n); for (i = n-1; i> 0; i--) { swap(a[0],a[i]); XXXXXXXXXXXXX; } } typedef void(*SortFunc)(int[], int); void testSort(SortFunc sortFunc,int n = 10){ DWORD start, end; int* a = new int[n]; randomAssign(a,n); if(n < 20) print("排序前",a,n); start = GetTickCount(); sortFunc(a,n); end = GetTickCount(); if(n < 20) print("排序后",a,n); cout<<"花费时间="<<end - start<<"毫秒"<<endl<<endl; delete [] a; } int main(){ testSort(bubbleSort,10); testSort(quickSort,10); testSort(heapSort,10); return 1; }
时间: 2023-11-22 11:54:06 浏览: 30
冒泡排序、快速排序和堆排序的代码已经提供,下面是完整的代码:
```c++
#include <iostream>
#include <cstdlib>
#include <Windows.h>
#include <ctime>
using namespace std;
void randomAssign(int a[], int n) {
srand(time(0));
for (int i = 0; i < n; i++)
a[i] = rand() % n + 1;
}
void print(const char* str, int a[], int n) {
cout << str << "=";
for (int i = 0; i < n; i++)
cout << a[i] << " ";
cout << endl;
}
void bubbleSort(int a[], int n) {
for (int i = n; i >= 1; i--)
for (int j = 1; j < i; j++)
if (a[j + 1] > a[j])
swap(a[j + 1], a[j]);
}
int onePartition(int a[], int left, int right) {
int pivot = a[left];
int i = left;
int j = right;
while (i < j) {
while (a[j] >= pivot && i < j)
j--;
if (i < j)
a[i++] = a[j];
while (a[i] <= pivot && i < j)
i++;
if (i < j)
a[j--] = a[i];
}
a[i] = pivot;
return i;
}
void quickSort(int a[], int left, int right) {
if (left >= right)
return;
int pos = onePartition(a, left, right);
quickSort(a, left, pos - 1);
quickSort(a, pos + 1, right);
}
void quickSort(int a[], int n) {
quickSort(a, 0, n - 1);
}
void sift(int a[], int pos, int n) { // n表示长度
int parent = pos;
int son = parent * 2 + 1;
int tmp = a[parent];
while (son <= n - 1) { // 有效下标 0...n-1
if (son + 1 <= n - 1 && a[son] < a[son + 1])
son++;
if (tmp >= a[son])
break;
else {
a[parent] = a[son];
parent = son;
son = parent * 2 + 1;
}
}
a[parent] = tmp;
}
void heapSort(int a[], int n) {
int i = 0;
for (i = n / 2 - 1; i >= 0; i--) //从最后一个非叶结点开始
sift(a, i, n);
for (i = n - 1; i > 0; i--) {
swap(a[0], a[i]);
sift(a, 0, i);
}
}
typedef void(*SortFunc)(int[], int);
void testSort(SortFunc sortFunc, int n = 10) {
DWORD start, end;
int* a = new int[n];
randomAssign(a, n);
if (n < 20)
print("排序前", a, n);
start = GetTickCount();
sortFunc(a, n);
end = GetTickCount();
if (n < 20)
print("排序后", a, n);
cout << "花费时间=" << end - start << "毫秒" << endl << endl;
delete[] a;
}
int main() {
testSort(bubbleSort, 10);
testSort(quickSort, 10);
testSort(heapSort, 10);
return 1;
}
```
需要注意的是:
1. 在快速排序的 onePartition 函数中,i 和 j 的初始值应该分别为 left 和 right,而不是 left + 1 和 right - 1。这是因为在 while 循环中,i 和 j 分别向中间移动,不会越界。
2. 在堆排序的 sift 函数中,比较的应该是 tmp 和 a[son],而不是 a[parent] 和 a[son]。
3. 在堆排序的循环中,交换 a[0] 和 a[i] 后,应该对 a[0] 进行 sift 调整,而不是对 a[i] 进行 sift 调整。因为 a[i] 已经排好序了,不需要再调整。
相关推荐
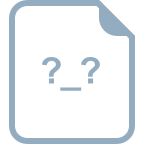














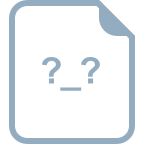