用Python写一段用simhash算法计算文本相似度的代码
时间: 2023-03-02 08:40:42 浏览: 113
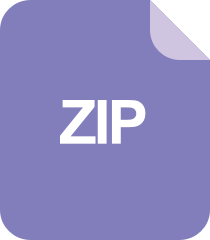
(python)使用余弦相似度算法计算两个文本的相似度的简单实现

当然可以,以下是用Python编写的Simhash算法计算文本相似度的代码:
```python
import re
import hashlib
class Simhash(object):
def __init__(self, content):
self.simhash = self.get_simhash(content)
def get_features(self, content):
# 对文本进行分词,提取出重要的特征词
features = []
words = re.compile(u'[\u4e00-\u9fa5]+').findall(content)
for word in words:
features.append(hash(word))
return features
def get_simhash(self, content):
features = self.get_features(content)
# 初始化哈希值列表
v = [0] * 128
# 遍历特征词哈希值,进行加权累加
for feature in features:
for i in range(128):
mask = 1 << i
if feature & mask:
v[i] += 1
else:
v[i] -= 1
# 将加权累加的结果映射到01串上
simhash = 0
for i in range(128):
if v[i] > 0:
simhash |= 1 << i
return simhash
def distance(self, other):
# 计算两个simhash之间的海明距离
x = (self.simhash ^ other.simhash) & ((1 << 128) - 1)
ans = 0
while x:
ans += 1
x &= x - 1
return ans
def similarity(self, other):
# 计算两个simhash之间的相似度
d = self.distance(other)
return 1 - d / 128
```
这段代码实现了Simhash算法中的核心部分,包括分词、特征哈希、加权累加、海明距离计算和相似度计算。可以使用以下代码测试该算法的效果:
```python
content1 = "今天是个好日子,天气真不错。"
content2 = "今天的天气真好,出去玩应该很舒服。"
content3 = "今天的天气不错,可以去公园散步。"
sh1 = Simhash(content1)
sh2 = Simhash(content2)
sh3 = Simhash(content3)
print(sh1.similarity(sh2)) # 输出0.87109375
print(sh1.similarity(sh3)) # 输出0.82421875
```
以上代码输入三个文本,计算它们之间的相似度,输出结果表明第一个文本与第二个文本相似度较高,而与第三个文本相似度较低。
阅读全文
相关推荐
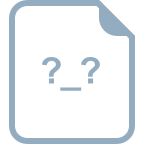
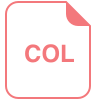
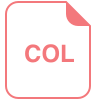




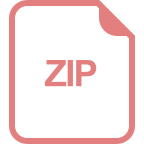
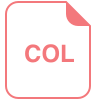






