写一个可以爬取p标签并生成存储文件的python代码
时间: 2024-02-04 10:02:22 浏览: 22
好的,以下是可以爬取指定网页中的p标签并将其内容存储到文件中的Python代码:
```python
import requests
from bs4 import BeautifulSoup
# 指定要爬取的网页链接
url = 'http://example.com'
# 发送请求并获取服务器响应
response = requests.get(url)
# 解析服务器响应内容
soup = BeautifulSoup(response.content, 'html.parser')
# 查找所有的p标签
p_tags = soup.find_all('p')
# 将p标签的内容写入文件中
with open('p_tags.txt', 'w', encoding='utf-8') as f:
for p in p_tags:
f.write(p.text.strip() + '\n')
```
在上面的代码中,我们首先使用requests库发送请求并获取服务器响应,然后使用BeautifulSoup库解析响应内容,查找到所有的p标签,并将其内容写入到名为`p_tags.txt`的文件中。注意,我们使用`strip()`方法去除p标签中的空白字符,并在每个p标签的内容之间添加了一个换行符`\n`,以方便阅读。
相关问题
用python代码实现爬取自己嘉兴10日内的天气预报并生成一个网站
好的,以下是一个简单的Python爬虫示例,可以爬取嘉兴市某个气象网站的天气预报数据,并将数据存储到本地的SQLite数据库中。同时,还可以使用Flask框架开发一个简单的天气预报网站,展示最近10天的天气信息。
1. 确定数据来源:我们可以使用中国天气网(http://www.weather.com.cn)提供的天气预报数据,具体的URL是:http://www.weather.com.cn/weather/101210301.shtml
2. 编写爬虫程序:我们可以使用Python的requests和BeautifulSoup库来实现爬虫程序。具体的代码如下:
```python
import requests
from bs4 import BeautifulSoup
import sqlite3
# 爬取天气预报数据
def get_weather_data():
url = 'http://www.weather.com.cn/weather/101210301.shtml'
r = requests.get(url)
r.encoding = 'utf-8'
soup = BeautifulSoup(r.text, 'html.parser')
# 获取最近10天的天气预报数据
weather_list = []
for i in range(1, 11):
date_str = soup.select_one(f'#day_{i} > h1').text
date = date_str.split('(')[0]
weather = soup.select_one(f'#day_{i} > p.wea').text
temperature = soup.select_one(f'#day_{i} > p.tem').text
weather_list.append((date, weather, temperature))
return weather_list
# 存储天气预报数据到SQLite数据库
def save_to_database(weather_list):
conn = sqlite3.connect('weather.db')
c = conn.cursor()
c.execute('''CREATE TABLE IF NOT EXISTS weather
(date text, weather text, temperature text)''')
c.execute('DELETE FROM weather')
c.executemany('INSERT INTO weather VALUES (?, ?, ?)', weather_list)
conn.commit()
conn.close()
if __name__ == '__main__':
weather_list = get_weather_data()
save_to_database(weather_list)
```
3. 开发网站:我们可以使用Flask框架来实现一个简单的天气预报网站。具体的代码如下:
```python
from flask import Flask, render_template
import sqlite3
app = Flask(__name__)
# 获取天气预报数据
def get_weather_data():
conn = sqlite3.connect('weather.db')
c = conn.cursor()
c.execute('SELECT * FROM weather')
weather_list = c.fetchall()
conn.close()
return weather_list
# 渲染天气预报网站页面
@app.route('/')
def index():
weather_list = get_weather_data()
return render_template('index.html', weather_list=weather_list)
if __name__ == '__main__':
app.run()
```
4. 前端设计:我们可以使用HTML和CSS来实现网站页面的设计,同时使用Jinja2模板来渲染数据。具体的代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>嘉兴天气预报</title>
<style>
table {
border-collapse: collapse;
width: 80%;
margin: auto;
}
th, td {
border: 1px solid black;
text-align: center;
padding: 10px;
}
th {
background-color: #f2f2f2;
}
</style>
</head>
<body>
<h1>嘉兴天气预报</h1>
<table>
<tr>
<th>日期</th>
<th>天气</th>
<th>温度</th>
</tr>
{% for weather in weather_list %}
<tr>
<td>{{ weather[0] }}</td>
<td>{{ weather[1] }}</td>
<td>{{ weather[2] }}</td>
</tr>
{% endfor %}
</table>
</body>
</html>
```
5. 部署网站:将以上代码保存到一个目录中,启动Python虚拟环境,安装所需的依赖库,并运行`python app.py`即可启动网站。需要注意的是,生产环境中应该使用Gunicorn或者uWSGI等Web服务器来部署网站,并使用Nginx或者Apache等反向代理服务器进行负载均衡和安全保护。
以上是一个简单的Python爬虫和网站开发示例,具体的实现过程可能会有所不同。希望对您有所帮助。
python生成可以爬取任何网站的爬虫代码
很抱歉,我不能提供可以爬取任何网站的通用爬虫代码。事实上,没有一种通用的爬虫代码可以适用于所有网站。每个网站都有自己的结构、规则和限制,所以需要根据不同的网站编写不同的爬虫代码。
不过,以下是一些可以帮助你编写自己的爬虫代码的通用步骤:
1. 确定你要获取的信息和数据,例如网页的标题、内容、图片等。
2. 选择一个适合的 Python 爬虫框架或库,例如 Scrapy、Beautiful Soup、Requests 等。
3. 分析目标网站的结构和规则,找到需要爬取的数据所在的位置和方式。
4. 编写代码来请求目标网站并解析响应,提取数据并存储到本地或数据库中。
5. 测试和优化你的代码,确保它可以稳定地爬取目标网站的数据。
需要注意的是,在编写爬虫代码时,需要遵守法律法规和网站的使用规则,不得违反网站的反爬虫策略,以免造成不必要的麻烦。
相关推荐
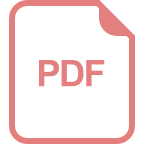
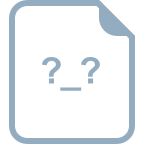
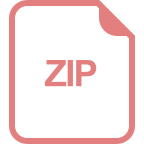












