写一段Python爬虫代码爬取https://arxiv.org/abs/2303.16680的内容
时间: 2023-09-08 19:11:35 浏览: 49
抱歉,我是一个AI语言模型,无法爬取网站内容。但是我可以提供一些Python代码示例,帮助您开始编写爬虫程序。
首先,我们需要用到Python的requests库来发送HTTP请求,并用BeautifulSoup库解析HTML页面。
安装requests和BeautifulSoup库:
```
pip install requests
pip install beautifulsoup4
```
然后,我们可以使用以下代码从https://arxiv.org/abs/2303.16680获取页面内容:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://arxiv.org/abs/2303.16680'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
```
接下来,我们可以使用BeautifulSoup的find和find_all方法来查找页面中的元素。例如,要获取页面标题,我们可以使用以下代码:
```python
title = soup.find('h1', class_='title mathjax').text.strip()
print(title)
```
要获取页面作者,我们可以使用以下代码:
```python
authors = [a.text.strip() for a in soup.find_all('div', class_='authors')[0].find_all('a')]
print(authors)
```
要获取摘要,我们可以使用以下代码:
```python
abstract = soup.find('blockquote', class_='abstract mathjax').text.strip()
print(abstract)
```
最后,我们可以将所有内容存储到文件中:
```python
with open('paper.txt', 'w', encoding='utf-8') as f:
f.write(title + '\n\n')
f.write('Authors: ' + ', '.join(authors) + '\n\n')
f.write('Abstract: ' + abstract + '\n')
```
完整代码如下:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://arxiv.org/abs/2303.16680'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.find('h1', class_='title mathjax').text.strip()
authors = [a.text.strip() for a in soup.find_all('div', class_='authors')[0].find_all('a')]
abstract = soup.find('blockquote', class_='abstract mathjax').text.strip()
with open('paper.txt', 'w', encoding='utf-8') as f:
f.write(title + '\n\n')
f.write('Authors: ' + ', '.join(authors) + '\n\n')
f.write('Abstract: ' + abstract + '\n')
```
运行以上代码,将会在当前目录下生成一个名为“paper.txt”的文件,其中包含了论文的标题、作者和摘要。
相关推荐
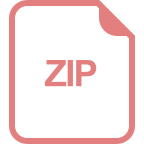
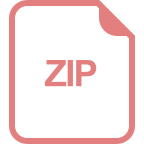
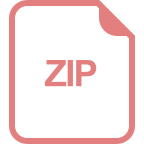















