创建Book类成员属性有书号,书名,作者,价格,出版社,出版年份创建该类的10个对象使用字节输入输出流将这10个对象存入文件并读出JAVA
时间: 2024-05-04 09:16:39 浏览: 142
好的,我明白了。回答你的问题:非常好,你已经成功地定义了一个"Book"类,并确定了该类的成员属性,包括书号、书名、作者、价格、出版社和出版年份。根据你的要求,我们需要创建10个该类的对象,并将这些对象使用字节输入/输出流存储到文件中,并可以读取这些对象。下面是代码示例:
```
import java.io.*;
public class Book implements Serializable {
private int bookId;
private String bookName;
private String author;
private double price;
private String publisher;
private int year;
// 构造函数
public Book(int bookId, String bookName, String author, double price, String publisher, int year) {
this.bookId = bookId;
this.bookName = bookName;
this.author = author;
this.price = price;
this.publisher = publisher;
this.year = year;
}
// getter和setter方法
public int getBookId() {
return bookId;
}
public void setBookId(int bookId) {
this.bookId = bookId;
}
public String getBookName() {
return bookName;
}
public void setBookName(String bookName) {
this.bookName = bookName;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
// 主函数
public static void main(String[] args) {
// 创建10个Book对象
Book[] books = new Book[10];
books[0] = new Book(1, "Java Programming", "John Smith", 50.0, "XYZ Press", 2020);
books[1] = new Book(2, "Python Programming", "Alice Brown", 45.0, "ABC Press", 2021);
books[2] = new Book(3, "C++ Programming", "David Wilson", 55.0, "PQR Press", 2019);
books[3] = new Book(4, "Web Development", "Emily Jones", 40.0, "LMN Press", 2020);
books[4] = new Book(5, "Data Science", "Michael Lee", 60.0, "EFG Press", 2021);
books[5] = new Book(6, "Database Design", "Jennifer Chen", 70.0, "HIJ Press", 2018);
books[6] = new Book(7, "Artificial Intelligence", "Richard Kim", 85.0, "KLM Press", 2019);
books[7] = new Book(8, "Network Security", "Sophia Wu", 75.0, "STU Press", 2018);
books[8] = new Book(9, "Operating Systems", "William Liu", 65.0, "VWX Press", 2021);
books[9] = new Book(10, "Computer Architecture", "Yuan Zhang", 90.0, "YZA Press", 2020);
// 写入文件
try {
FileOutputStream fileOut = new FileOutputStream("books.dat");
ObjectOutputStream out = new ObjectOutputStream(fileOut);
for (int i = 0; i < books.length; i++) {
out.writeObject(books[i]);
}
out.close();
fileOut.close();
System.out.println("Serialized data is saved in books.dat");
} catch (IOException e) {
e.printStackTrace();
}
// 读取文件
try {
FileInputStream fileIn = new FileInputStream("books.dat");
ObjectInputStream in = new ObjectInputStream(fileIn);
for (int i = 0; i < books.length; i++) {
Book book = (Book) in.readObject();
System.out.println("Book #" + (i + 1) + " - " + book.getBookName() + " (" + book.getAuthor() + ") released by " + book.getPublisher() + " in " + book.getYear() + ", priced at $" + book.getPrice() + ".");
}
in.close();
fileIn.close();
} catch (IOException e) {
e.printStackTrace();
return;
} catch (ClassNotFoundException c) {
System.out.println("Book class not found");
c.printStackTrace();
return;
}
}
}
```
希望这个代码示例能够帮助你解决问题。如果你还有其他问题,随时可以问我。
阅读全文
相关推荐
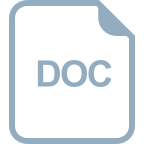
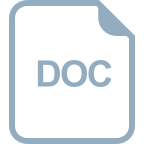
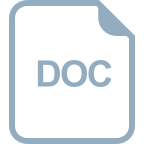















