用python写一段Canny算子的边缘检测程序
时间: 2023-06-04 21:02:13 浏览: 44
好的,以下是用Python实现Canny算子的边缘检测程序:
```python
import cv2
# 读取图像
img = cv2.imread('test.jpg', cv2.IMREAD_GRAYSCALE)
# Canny算子边缘检测
edges = cv2.Canny(img, 100, 200)
# 显示结果
cv2.imshow('output', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
其中,`cv2.imread()`函数用来读取图像,`cv2.IMREAD_GRAYSCALE`表示以灰度模式读取。
`cv2.Canny()`函数用来进行Canny算子边缘检测,其中第二个和第三个参数分别为低阈值和高阈值,可以根据具体需求进行调整。
最后,使用`cv2.imshow()`函数显示结果,`cv2.waitKey()`等待用户按键关闭窗口,`cv2.destroyAllWindows()`释放窗口。
相关问题
帮我写一段采用 OTSU 算法改进的Canny 算子边缘检测的基于python&opencv的代码
当然可以,以下是基于Python的OTSU算法改进版Canny边缘检测代码:
```python
import cv2
import numpy as np
def otsu_threshold(img):
# OTSU二值化处理
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
ret,thresh = cv2.threshold(gray_img,0,255,cv2.THRESH_BINARY+cv2.THRESH_OTSU)
return thresh
def canny_detector(img, thresh1=100, thresh2=200, apertureSize=3, L2gradient=True):
# Canny边缘检测
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray_img, thresh1, thresh2, apertureSize=apertureSize, L2gradient=L2gradient)
return edges
# 加载图片
img = cv2.imread('test.jpg')
# OTSU处理
otsu_img = otsu_threshold(img)
# Canny检测
canny_img = canny_detector(img)
# 比较Canny和OTSU+Canny结果,选择更优的结果
result_img = cv2.bitwise_or(otsu_img, canny_img)
# 显示结果
cv2.imshow("Result", result_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这里,我们首先对输入图像进行了OTSU二值化处理,然后使用OTSU二值化后的结果和Canny检测的结果进行了逐像素逻辑或运算,得到最终结果。
这种改进的方法可以弥补Canny算法在某些情况下对光照和噪声敏感的问题,从而提高边缘检测的准确性。
Canny算子python实现
可以使用OpenCV库来实现Canny算子的Python代码,下面是一个示例:
```
import cv2
# 读取图像
img = cv2.imread('image.jpg')
# 灰度化处理
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Canny边缘检测
edges = cv2.Canny(gray, 100, 200)
# 显示处理后图像
cv2.imshow('Canny', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码通过OpenCV库实现了Canny算子的Python代码,将指定图像转换为灰度图后,使用Canny函数进行边缘检测,并将结果显示在屏幕上。
相关推荐
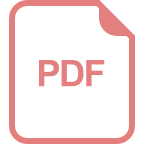
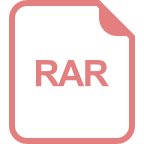












